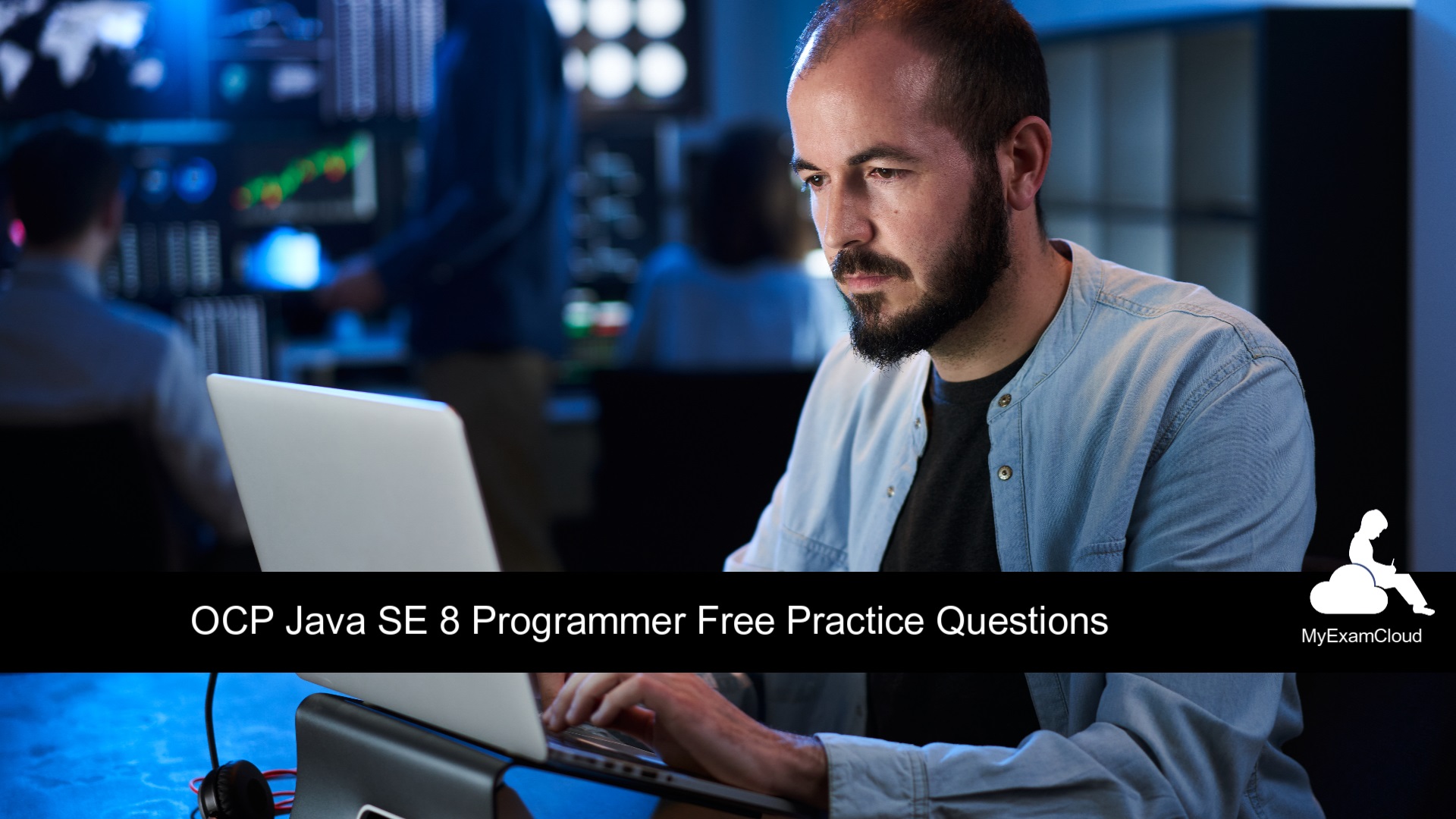
OCPJP 8 Practice Free Practice Questions
The following practice questions are designed to help you prepare for the Oracle Certified Associate Java Programmer 8 certification exam. This exam assesses your understanding and proficiency in programming concepts and the Java language. These practice questions are based on the OCPJP 8 Practice Tests Study Plan provided by MyExamCloud. The study plan is a comprehensive guide to help individuals prepare for the certification exam. By completing these practice questions, you can become familiar with the exam format and content, and improve your chances of success.
Read the explanations for the following questions from MyExamCloud OCPJP 8 Practice Tests Study Plan by taking Free 1Z0-809 Java SE 8 Programmer II Practice Mock Exam. The explanations are brief and can help review key concepts and prepare for the exam.
Topic:Java Class Design
QUESTION:1
Given :
public class MyExamCloud {
static {
x = 15;
}
MyExamCloud() {
x = 5;
}
static int x = 0;
public static void main(String[] args) {
MyExamCloud mec = new MyExamCloud();
System.out.println(x);
}
static {
x = 10;
}
}
What is the result ?
A:0
B:5
C:10
D:15
E:Compilation fails.
ANSWER: Choice B
Topic:Generics and Collections
QUESTION:2
Given
import java.util.stream.Stream;
public class MyExamCloud {
public static void main(String[] args) {
Stream < Integer > ints = Stream.of(1, 2, 3);
ints.forEach((Integer i) -> {
System.out.print(i * 2);
});
ints.forEach(System.out::print);
}
}
What is the output?
A:123246
B:246123
C:246 followed by an exception.
D:No output
E:Compilation fails.
ANSWER: Choice C
Topic:Exceptions and Assertions
QUESTION:3
Given
public class MyExamCloud {
public static void main(String args[]) {
double d = 0;
try {
d = Double.parseDouble("6d");
}
System.out.print(d);
catch(NumberFormatException e) {
System.out.print("Number Format Exception");
}
}
}
What is the output?
A:6
B:6d
C:6.0
D:Number Format Exception
E:Compilation fails.
ANSWER: Choice E
Topic:Java Concurrency
QUESTION:4
Assume that there are two private integer variables called i and j in your class.
Which of the following will prevent the memory consistency error?
A:
public double divide() {
synchronized(I, j) {
return (i / j);
}
}
public void set(int i, int j) {
synchronized(I, j) {
this.i = I;
this.j = j;
}
}
B:
public double divide() {
synchronized(i) {
return (i / j);
}
}
public void set(int i, int j) {
synchronized(j) {
this.i = I;
this.j = j;
}
}
C:
public synchronized(this) double divide() {
return (i / j);
}
public synchronized(this) void set(int i, int j) {
this.i = I;
this.j = j;
}
D:
public double divide() {
synchronized {
return (i / j);
}
}
public void set(int i, int j) {
synchronized {
this.i = I;
this.j = j;
}
}
E:
public double divide() {
synchronized(this) {
return (i / j);
}
}
public void set(int i, int j) {
synchronized(this) {
this.i = I;
this.j = j;
}
}
ANSWER: Choice E
Topic:Java Stream API
QUESTION:5
Given
import java.util.Optional;
public class MyExamCloud {
public static void main(String[] args) {
String[] in = new String[3];
String op1 = Optional.of(in [2]).orElse("Empty");
System.out.println(op1);
}
}
What is the output?
A:Empty
B:Null
C:NullPointerException
D:NoSuchElementException
E:Compilation fails.
ANSWER: Choice C
Topic:Java Concurrency
QUESTION:Which of the followings is true?
A:The ForkJoinTask class can't return a result.
B:The ForkJoinTask is a sub class of RecursiveTask class.
C:The ForkJoinTask class has only the "fork()" and "join()" methods.
D:None of above.
ANSWER: Choice D
Topic:Generics and Collections
QUESTION:6
Given:
import java.util.Comparator;
public class MyExamCloud {
public static void main(String[] args) {
Comparator comp = Comparator.nullsLast(Integer::compare);
System.out.println(comp.compare(null, 10));
}
}
What is the output?
A:-1
B:1
C:0
D:A NullPointerException.
E:Compile Error at line 6
ANSWER: Choice B
Topic:Exceptions and Assertions
QUESTION:7
Given :
public class MyExamCloud {
public static void main(String[] args) {
new MyExamCloud().go();
}
void go() {
System.out.print("A ");
try {
run(0);
System.out.print("B ");
} catch(Exception e) {
System.out.print("C ");
} finally {
System.out.print("D ");
}
}
void run(int i) {
try {
System.out.print("E ");
int x = 5 / i;
System.out.print("F ");
} catch(NumberFormatException e2) {
System.out.print("G ");
} finally {
System.out.print("H ");
}
}
}
What will be the result?
A:A E G H C D
B:A E H B C D
C:A E H C D
D:A E H C D followed by an uncaught exception.
E:Compilation fails
ANSWER: Choice C
Topic:Exceptions and Assertions
QUESTION:8
Given the following set of classes:
class Exception A extends Exception {}
class Exception B extends A {}
class Exception C extends B {}
What is the correct sequence of catch blocks for the following try block?
try {
// codes
}
A:Catch Exception, A, B,C
B:Catch Exception, C, B, and A
C:Catch A, B, C, and Exception
D:Catch C, B ,A and Exception
E:Any order.
ANSWER: Choice D
Topic:Building Database Applications with JDBC
QUESTION:9
Which of the following can be used to execute stored procedures that may contain both input and output parameters?
A:statement
B:ResultSet
C:PreparedStatement
D:CallableStatement
E:None of above.
ANSWER: Choice D
Topic:Building Database Applications with JDBC
QUESTION:10
Given
import java.sql. * ;
public class MyExamCloud {
public static void main(String[] args) throws ClassNotFoundException,
SQLException {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = null;
Statement stmt = null;
String sql;
conn = DriverManager.getConnection("jdbc:mysql://localhost/db?" + "user=root&password=Admin123");
stmt = conn.createStatement();
sql = "DESC persons";
ResultSet rs = stmt.executeQuery(sql);
rs.first();
rs.next();
System.out.println(rs.getString(1));
}
}
Consider following table structure for the person table.
What is the output?
A:LastName
B:PersonID
C:varchar(255)
D:YES
E:Compilation fails.
ANSWER: Choice A
Topic:Building Database Applications with JDBC
QUESTION:11
Given
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class MyExamCloud {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = null;
Statement stmt = null;
String sql;
conn = DriverManager.getConnection("jdbc:mysql://localhost/?"+ "user=root&password=Admin123");
stmt = conn.createStatement();
sql = "create database db";
System.out.println(stmt.executeQuery(sql));
}
}
What is the output?
A:True will be printed and a new database with name "db" will be created.
B:False will be printed and a new database with name "db" will be created.
C:False will be printed but a new database won't be created.
D:An Exception.
E:Compilation fails.
ANSWER: Choice D
Topic:Use Java SE 8 Date/Time API
QUESTION:12
Given:
import java.time.LocalDate;
import java.time.Period;
public class MyExamCloud {
public static void main(String[] args) {
Period period = Period.of(1, 2, 1);
LocalDate loc = LocalDate.of(2015, 1, 1);
loc = loc.plusDays(period.getDays());
System.out.println(loc);
}
}
What is the result?
A:2015-01-01
B:2015-01-02
C:2015-01-03
D:2015-01-04
E:Compilation fails.
ANSWER: Choice B
Topic:Use Java SE 8 Date/Time API
QUESTION:13
Given:
import java.time.Duration;
import java.time.LocalDate;
public class MyExamCloud {
public static void main(String[] args) {
LocalDate ld0 = LocalDate.of(2015, 1, 27);
LocalDate ld1 = LocalDate.of(2015, 1, 31);
Duration due = Duration.between(ld0, ld1);
System.out.println(due);
}
}
What is the result?
A:PT96H
B:PT4D
C:PT3D
D:An Exception.
E:Compilation fails.
ANSWER: Choice D
Topic:Use Java SE 8 Date/Time API
QUESTION:14
Which of the following can be used to create a MonthDay instance?
A:new MonthDay(10,28);
B:MonthDay.get(10,28);
C:MonthDay.of(10,28);
D:MonthDay.of(28,Month.OCTOBER);
E:None of above.
ANSWER: Choice C
Topic:Generics and Collections
QUESTION:15
Given:
import java.util.Set;
import java.util.TreeSet;
public class MyExamCloud {
public static void main(String[] args) {
Set set = new TreeSet < > ();
set.add("A");
set.add("D");
set.pollFirst();
System.out.println(set.ceiling("C"));
}
}
What is the result?
A:D
B:A
C:null
D:An Exception.
E:Compilation fails.
ANSWER: Choice E
Topic:Generics and Collections
QUESTION:16
Given:
import java.util.Map;
import java.util.TreeMap;
import java.util.HashMap;
public class MyExamCloud {
public static void main(String[] args) {
Map map = new HashMap < > ();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
map.put("Four", 4);
TreeMap tmap = new TreeMap(map);
System.out.println(tmap.ceilingKey("O"));
}
}
What is the result?
A:One=1
B:One
C:2
D:Two
E:Compilation fails.
ANSWER: Choice B
Topic:Generics and Collections
QUESTION:17
Given:
import java.util.ArrayDeque;
public class MyExamCloud {
public static void main(String[] args) {
ArrayDeque ad = new ArrayDeque < > ();
ad.add(6);
ad.add(2);
ad.offerLast(3);
ad.offer(4);
ad.poll();
System.out.println(ad);
}
}
What is the result?
A:[6, 2, 1]
B:[6, 2, 3]
C:[2, 3, 4]
D:[6, 2, 3, 4]
E:Compilation fails.
ANSWER: Choice C
Topic:Generics and Collections
QUESTION:18
Which of the following lambda can be used for String typed Comparable?
A:s -> return s.length()
B:s -> s.isEmpty();
C:s -> s;
D:s -> s.hashCode();
E:None of above.
ANSWER: Choice D
Topic:Java I/O Fundamentals
QUESTION:19
Given:
import java.io.Console;
public class MyExamCloud {
public static void main(String[] args) {
Console c = System.console();
_ _ _ _ _ _ _ _ oldPassword = c.readPassword("password: ");
}
}
Which of the following can be used to fill the blank?
A:String
B:String[]
C:char[]
D:Console
E:None of above.
ANSWER: Choice C
Topic:Java I/O Fundamentals
QUESTION:20
Given:
import java.io.FileReader;
import java.io.IOException;
public class MyExamCloud {
public static void main(String[] args) throws IOException {
FileReader fr = new FileReader("new.txt");
System.out.println(fr.read());
fr.close();
}
}
Content of the new.txt : ABCD
What is the output?
A:ABCD
B:A
C:65
D:Compilation fails due to error at line 6.
E:Compilation fails due multiple errors.
ANSWER: Choice C
Topic:Java File I/O (NIO.2)
QUESTION:21
Given:
import java.io.IOException;
import java.nio.file.Path;
import java.nio.file.Paths;
public class MyExamCloud {
public static void main(String[] args) throws IOException {
Path p1 = Paths.get("in\\new");
Path p2 = Paths.get("file.txt");
System.out.println(p1.resolve(p2));
}
}
What is the output?
A:in\new\file.txt
B:new\file.txt
C:file.txt
D:An Exception.
E:Compilation fails.
ANSWER: Choice A
Topic:Java File I/O (NIO.2)
QUESTION:22
Given:
import java.io.IOException;
import java.nio.*;
import java.nio.file.*;
import java.util.*;
import java.util.stream.*;
public class MyExamCloud {
public static void main(String[] args) {
try {
Path path = Paths.get("input.txt");
Stream < String > stream = Files.readAllLines(path);
stream.limit(1).forEach(System.out::print);
} catch (IOException ex) {
System.out.println("Error!");
}
}
}
Content of the input.txt :
C
B
A
What is the output?
A:CBA
B:A
C:C
D:Error!
E:Compilation fails.
ANSWER: Choice E
Topic:Java File I/O (NIO.2)
QUESTION:23
Given:
import java.util.stream.*;
public class MyExamCloud {
public static void main(String[] args) {
Stream ints = Stream.of(3, 6, 0, 4);
ints.sorted().peek(System.out::print).findFirst();
}
}
What is the output?
A:No output.
B:3604
C:3
D:0
E:Compilation fails.
ANSWER: Choice D
Topic:Java Stream API
QUESTION:24
Given:
import java.util.stream.Stream;
public class MyExamCloud {
public static void main(String[] args) {
Stream ints = Stream.of(1, 2, 3);
int i = ints.skip(1).limit(1).count();
System.out.println(i);
}
}
What is the output?
A:3
B:1
C:2
D:0
E:Compilation fails.
ANSWER: Choice E
Topic:Java Class Design
QUESTION:25
Given:
interface I {}
abstract class A implements I {}
class B extends A {}
public class MyExamCloud {
public static void main(String[] args) {
I i = new B();
A a = new B();
boolean t1 = i instanceof I, t2 = a instanceof I;
System.out.print(t1 + " " + t2);
}
}
What is the result?
A:The output will be true true
B:The output will be true false
C:The output will be false false
D:Compilation fails due to error at line 11.
E:Compilation fails due to multiple errors.
ANSWER: Choice A
Topic:Building Database Applications with JDBC
QUESTION:26
Consider following steps
- Create a statement.
- Establishing a connection.
- Execute the query.
- Close the connection
- Process the ResultSet object.
What is the correct order of above steps to process any SQL statement with JDBC?
A:1,2,3,4,5
B:2,1,3,5,4
C:1,2,3,5,4
D:2,1,3,4,5
E:2,5,1,3,4
ANSWER: Choice B
Topic:Advanced Class Design
QUESTION:27
Which of following represents a correct abstract class?
A:abstract class A { public void meth() { } }
B:abstract class A{ public void meth(); }
C:class A{ public void meth(); }
D:final abstract class A{ public abstract void meth(); }
E:None of above.
ANSWER: Choice A
Topic:Localization
QUESTION:28
Given
import java.util. * ;
public class MyExamCloud {
public static void main(String args[]) {
Locale ENUS = new Locale.Builder().setLanguage("en").setRegion("US").build();
System.out.print(ENUS.getDisplayCountry(new Locale("fr")));
}
}
What is the output?
A:Code will print the word "English" in English language (English).
B:Code will print the word "english" in French language (anglais).
C:Code will print the word "anglais" in English language (English).
D:Code will print the word "french" in French language (french).
E:Code will print the word "United States" in French language (Etats-Unis).
ANSWER: Choice E
Author | JEE Ganesh | |
Published | 7 months ago | |
Category: | Java Certification | |
HashTags | #Java #JavaCertification |