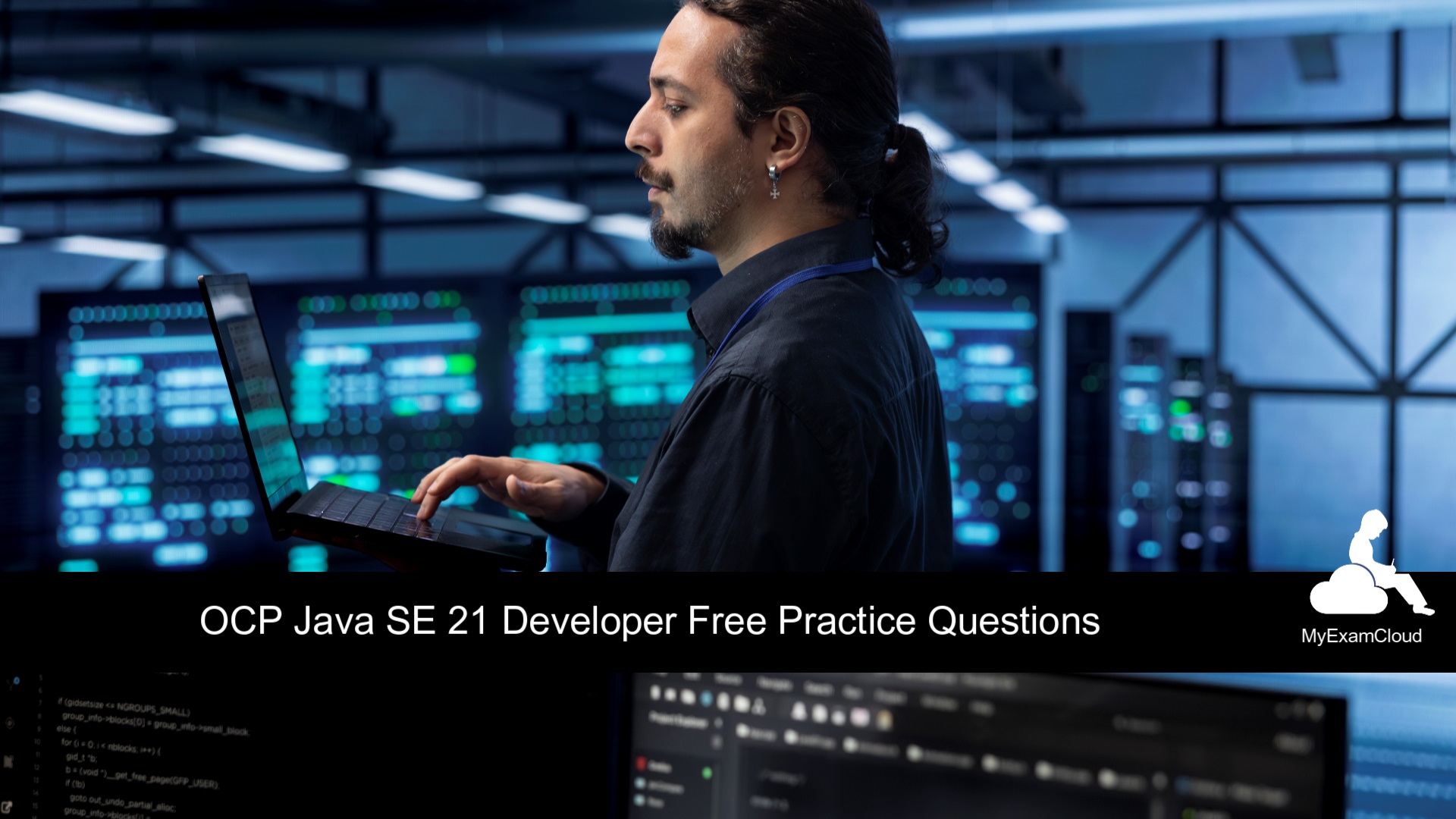
OCP Java 21 Developer 1Z0-830 Free Practice Questions
These practice questions are designed to assist you in your preparation for the Oracle Certified Professional: Java SE 21 Developer certification exam. This certification is intended for Java developers to validate their skills and knowledge in Java Standard Edition (SE) 21. It serves as a benchmark for employers to evaluate a developer's proficiency in Java programming, including their ability to utilize modern features of Java SE 21.
These practice questions are formulated based on the 1Z0-830 Practice Tests Study Plan provided by MyExamCloud. This study plan is a comprehensive guide that covers all the necessary topics for the certification exam. By completing these practice questions, you can familiarize yourself with the exam format and content, and improve your chances of passing the exam.
For a better understanding of the questions, you can also refer to the explanations provided in the 1Z0-830 Java SE 21 Developer Free Practice Mock Exam. These explanations are concise yet helpful in reviewing key concepts and sharpening your skills for the actual exam.
Topic:Working with Streams and Lambda expressions
QUESTION: 1
Code:
1. import java.util.stream.DoubleStream;
2.
3. public class MyExamCloud {
4.
5. public static void main(String[] args){
6.
7. var doubles = DoubleStream.of(0.45,0.42,0.49);
8. System.out.print(doubles.filter(e -> e>0.45).count());
9. }
10. }
What is the output?
A:1
B:2
C:Compilation fails since we haven't imported predicate
D:Compilation fails due to error at line 7
E:Compilation fails due to error at line 8
ANSWER: Choice A
Topic:Packaging and Deploying Java Code
QUESTION: 2
Given that a class named com.myexamcloud.exam.Exam
is part of a module named com.myexamcloud.exam
packaged in myexam.jar
, which of the following commands can be used to execute this class?
(Assume that the jar file is located in the current directory.)
A:java --module-path myexam.jar --module com.myexamcloud.exam.Exam
B:java --module-path myexam.jar --module com.myexamcloud.exam com.myexamcloud.exam.Exam
C:java --module-path myexam.jar --module com.myexamcloud.exam --main-class com.myexamcloud.exam.Exam
D:java --module-path myexam.jar --module com.myexamcloud.exam/com.myexamcloud.exam.Exam
E:java --module-path . --module com.myexamcloud.exam/com.myexamcloud.exam.Exam
ANSWER: Choice D,Choice E
Topic:Manage concurrent code execution
QUESTION: 3
Consider the following code:
record Account(String accNumber, double balance) {
static java.util.concurrent.locks.ReentrantLock lock = new java.util.concurrent.locks.ReentrantLock();
public double withdraw(double amt) {
double latestBalance = 0;
try {
lock.lock();
if (balance > amt) latestBalance = balance - amt;
} finally {
lock.unlock();
}
return latestBalance;
}
}
What can be done to make the above code thread safe?
A:Change new ReentrantLock() to new ReentrantLock(true).
B:Make the lock variable as protected.
C:Make the lock variable protected, final, and static.
D:Move the call to lock.lock(); to before the try block.
E:Make the lock variable final.
F:No change is required.
G:Declare lock variable as private and final.
ANSWER: Choice G
Topic:Use Java I/O API
QUESTION: 4
Given:
import java.io.*;
class MyExamCloud {
public static void main(String[] args) throws Exception {
try (var bfr = new BufferedReader(new InputStreamReader(System.in))) {
System.out.println("Enter Your Name:");
var s = bfr.readLine();
System.out.println("Your Name is : " + s);
} catch (Exception e) {
e.printStackTrace();
}
}
}
What will be the output if the above code is executed using the following command:
java MyExamCloud Joe
A:Enter Your Name:
Your Name is: MyExamCloud Joe
B:An exception stack trace will be printed.
C:Enter Your Name:
Your Name is : null
D:Enter Your Name:
E:Enter Your Name:
Your Name is : Joe
ANSWER: Choice D
Topic:Controlling Program Flow
QUESTION: 5
Which of the following Java SE 21 code snippets compile without any error?
The variable obj is of type java.lang.Object.
A:
if (obj instanceof String name & name.length() > 10) {
var xPosition = 10;
var yPosition = 0;
}
B:
if (obj instanceof String name && name.length() > 10) {
var xPosition = 10;
var yPosition = 0;
}
C:
if (obj instanceof String name | name.length() > 10) {
var xPosition = 10;
var yPosition = 0;
}
D:
None of above
ANSWER: Choice B
Topic:Controlling Program Flow
QUESTION: 6
Code Given:
//Saved as MyExamCloud.java
class Account {}
class StandardAccount extends Account {}
class PremiumAccount extends Account {
int monthsRemaining() {
return 10;
}
}
public class MyExamCloud {
record Point(int i, int j) {}
static void testType(Object o) {
switch (o) {
case null -> System.out.println("null");
case String s -> System.out.println("String");
case StandardAccount a -> System.out.println("Account");
case PremiumAccount p -> System.out.println("PremiumAccount");
case int[] ia -> System.out.println("Array");
default -> System.out.println("Something else");
}
}
public static void main(String as[]) {
testType(new PremiumAccount());
testType(new StandardAccount());
testType(new Account());
testType(null);
testType("");
testType(new int[] {
1, 2, 3, 4, 5
});
}
}
What is not true about MyExamCloud.java when you compile and run the above code?
A:The code may print both Account and PremiumAccount
B:The code will never print Something else
C:The code may print both Array and Something else
D:The code may print null and String
ANSWER: Choice B
Topic:Controlling Program Flow
QUESTION: 7
Code:
class Exam {
public String toString() {
return "MyExamCloud Practice Exam";
}
}
class MyExamCloud {
public static void main(String as[]) {
Object o = new Exam();
switch (o) {
case null -> System.out.println("null");
case String s -> System.out.println("String");
case Exam e -> System.out.println(e.toString());
case int[] ia -> System.out.println("Array length" + ia.length);
default -> System.out.println("Something else");
}
}
}
What is the output?
A:@Exam
B:String
C:Array length 0
D:null
E:MyExamCloud Practice Exam
F:Something else
ANSWER: Choice E
Topic:Using Object-Oriented Concepts in Java
QUESTION: 8
Code Given:
record Customer(Account account, int customerId) implements java.io.Serializable { // Line 1
public int customerId() {
return this.customerId + 1; // Line 3
}
public void someMethod() { // Line 4
System.out.println("Some method");
}
public static void test() { // Line 5
}
static {
System.out.println("A static intilizer record "); // Line 6
}
record InnerRecord() {} // Line 7
}
What is true about Customer record class?
A:Compiler error at // Line 5, we cannot have static methods
B:Compiler error at // Line 6, we cannot have static intilizer blocks
C:This type of record class should be considered bad style
D:Compiler error // Line 4, we cannot have additional instance methods
E:Compiler error at // Line 3, customerId is private final
F:Compiler error at // Line 7, we cannot have inner records
ANSWER: Choice C
Topic:Handing date, time, text, numeric and boolean values
QUESTION: 9
Given the following options.
Which of the choices will fail to compile?
A:String myArticle =
"""
Java SE 21 is Good.
MyExamCloud Tests are realy useful
for latest Java Certifications. " """;
B:String type = ...
String code = String.format("""
public void print(%s o) {
System.out.println(Objects.toString(o));
}
""", type);
C:String code =
"""
String text = \"""
A text block inside a text block
\""";
""";
D:String myQuote =""" Pain is the healing process.
Failue is the success process.""";
ANSWER: Choice D
Topic:Handing date, time, text, numeric and boolean values
QUESTION: 10
Which of the following code correctly utilize Java Date/Time API for DST?
A:LocalDate date = LocalDate.now();
System.out.println(date);
B:Calendar cal = Calendar.getInstance();
TimeZone tz = TimeZone.getTimeZone("Europe/London");
cal.setTimeZone(tz);
C:Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy HH:mm:ss");
sdf.setTimeZone(TimeZone.getTimeZone("Europe/London"));
System.out.println(sdf.format(date));
D:LocalDateTime localDateTimeBeforeDST = LocalDateTime.now();
ZoneId uk = ZoneId.of("Europe/London");
ZonedDateTime zonedDateTimeBeforeDST = localDateTimeBeforeDST.atZone(uk);
ZonedDateTime zonedDateTimeAfterDST = zonedDateTimeBeforeDST.plus(10, ChronoUnit.DAYS);
ANSWER: Choice D
Topic:Using Object-Oriented Concepts in Java
QUESTION: 11
Given the following code:
record Line(int x, int y) {}
enum Color {
RED, GREEN, BLUE
}
record ColoredLine(Line l, Color c) {}
record Rectangle(ColoredLine top, ColoredLine bottom, ColoredLine left, ColoredLine right) {}
Which code can extract the color from the top line using Java 21 record patterns?
A:static void printTopColoredLine(Rectangle r) {
if (r instanceof Rectangle(ColoredLine(Point p, Color c),
ColoredLine bottom, ColoredLine left, ColoredLine right)) {
System.out.println(c);
}
}
B:static void printTopColoredLine(Rectangle r) {
if (r instanceof Rectangle(ColoredLine top, ColoredLine bottom, ColoredLine left, ColoredLine right)) {
System.out.println(top.c());
}
}
C:static void printTopColoredLine(Rectangle r) {
if (r instanceof Rectangle(ColoredLine top, ColoredLine bottom, ColoredLine left, ColoredLine right) r) {
System.out.println(r.top.c());
}
}
D:static void printTopColoredLine(Rectangle r) {
if (r instanceof Rectangle r) {
System.out.println(top.c());
}
}
E:static void printTopColoredLine(Rectangle r) {
if (r instanceof Rectangle r) {
System.out.println(r.top.c());
}
}
ANSWER: Choice A,Choice B
Topic:Manage concurrent code execution
QUESTION: 12
Which of the following options creates virtual threads?
A:Thread thread = new Thread().start(() -> System.out.println("My Virtual Thread"));
thread.join();
B:Thread thread = Thread.ofVirtual().start(() -> System.out.println("My Virtual Thread"));
thread.join();
C:Thread.Builder builder = Thread.ofPlatform().name("MyVirtualThread");
Runnable task = () -> {
System.out.println("Running...");
};
Thread t = builder.start(task);
System.out.println("Thread t name: " + t.getName());
t.join();
D:Thread.Builder builder = Thread.ofVirtual().name("MyVirtualThread");
Runnable task = () -> {
System.out.println("Running...");
};
Thread t = builder.start(task);
System.out.println("Thread t name: " + t.getName());
t.join();
ANSWER: Choice B,Choice D
Topic:Working with Arrays and Collections
QUESTION: 13
What is the output of the following code?
import java.util.*;
public class MyExamCloud {
public static void main(String[] args) {
var lst = new ArrayList<String>();
lst.addFirst("a1");
lst.add("a2");
lst.addLast("a3");
var x1 = Collections.binarySearch(lst, "a3");
System.out.println(x1);
Collections.sort(lst);
var x2 = Collections.binarySearch(lst, "a3");
System.out.println(x2);
var list2 = lst.reversed();
var x3 = Collections.binarySearch(list2, "a3");
System.out.println(x3);
var x4 = Collections.binarySearch(list2, "a0");
System.out.println(x4);
}
}
A:2
2
2
0
B:2
2
-4
-1
C:2
0
-2
-1
D:2
2
2
0
ANSWER: Choice B
Topic:Using Object-Oriented Concepts in Java
QUESTION: 14
What is the output of the following code?
// saved as MyExamCloud.java
sealed class Account permits BankAccount {
public void methodA() {
System.out.println("methodA");
}
}
sealed class BankAccount extends Account permits CreditAccount {
public void methodB() {
System.out.println("methodB");
}
}
non-sealed class CreditAccount extends BankAccount {
public void methodC() {
System.out.println("methodC");
}
}
public class MyExamCloud {
public static void main(String[] args) {
Account bA = new BankAccount();
Account cA = new CreditAccount();
if (cA instanceof BankAccount ba) {
ba.methodB();
if (ba instanceof CreditAccount ca) {
ca.methodC();
}
} else {
cA.methodA();
}
}
}
A:methodB
methodC
B:methodB
methodB
C:methodB
methodA
D:methodA
methodB
ANSWER: Choice A
Topic:Using Object-Oriented Concepts in Java
QUESTION: 15
What is the output of the following code?
import java.util.*;
public class MyExamCloud {
public enum AI_MODELS {
ML('A'), SL('B'), UL('C'), DL('D');
char c;
private AI_MODELS(char c) {
this.c = c;
}
}
public static void main(String[] args) {
Arrays.stream(AI_MODELS.values()).dropWhile(s -> s.equals(AI_MODELS.SL));
switch (AI_MODELS.valueOf("SL")) {
case ML -> System.out.println("Machine learning");
case SL -> System.out.println("Supervised learning");
case UL -> System.out.println("Unsupervised learning");
case DL -> System.out.println("Deep learning");
default -> System.out.println("Undefined AI Model");
}
}
}
A:Machine learning
B:Supervised learning
C:Unsupervised learning
D:Deep learning
E:Undefined AI Model
F:The code will not compile
ANSWER: Choice B
Topic:Use Java I/O API
QUESTION: 16
Given the following classes:
// saved as Course.java
import java.io.*;
public class Course implements Serializable {
private static float averagePrice = 9.99 f;
private String description;
private transient float price;
public Course(String description, float price) {
this.description = description;
this.price = price;
}
public void readObject(ObjectInputStream in ) throws IOException, ClassNotFoundException {
in .defaultReadObject();
price = averagePrice;
}
public String toString() {
return description + " " + price + " " + averagePrice;
}
}
// Saved as MyExamCloud.java
import java.io.*;
public class MyExamCloud {
public static void main(String[] args) {
Course c = new Course("Java 21 Practice Tests", 19.99f);
try {
try (ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("c.course"))) {
out.writeObject(c);
}
try (ObjectInputStream in = new ObjectInputStream(new FileInputStream("c.course"))) {
c = (Course) in .readObject();
}
} catch (Exception e) {
e.printStackTrace();
}
System.out.println(c);
}
}
What is the output?
A:Java 21 Practice Tests 9.99 9.99
B:Java 21 Practice Tests 19.99 19.99
C:Java 21 Practice Tests 0.0 0.0
D:An exception is produced at runtime
E:Compilation fails
F:Java 21 Practice Tests 0.0 9.99
ANSWER: Choice F
Topic:Using Object-Oriented Concepts in Java
QUESTION: 17
What is the output of the following code?
public class MyCalculator {
public void addAll(int a, int b) {
System.out.print(" A");
}
public void addAll(int a, float b) {
System.out.print(" B");
}
public void addAll(float a, float b) {
System.out.print(" C");
}
public void addAll(double...a) {
System.out.print(" D");
}
public static void main(String[] args) {
var mc = new MyCalculator();
mc.addAll(1, 2.5f);
mc.addAll(1, 2);
mc.addAll(1.5, 2.5);
}
}
A:B A C
B:D A D
C:B A D
D:D D D
ANSWER: Choice C
Topic:Working with Arrays and Collections
QUESTION: 18
What is the output of the following code?
import java.util.*;
public class MyExamCloud {
public String addCourse1(List<String> data) {
return data.parallelStream().reduce("J", (n, m) -> n + m, String::concat);
}
public String addCourse2(List<String> data) {
return data.parallelStream().reduce((l, p) -> l + p).get();
}
public static void main(String[] args) {
var mc = new MyExamCloud();
var list = List.of("Java", "Python");
var c1 = mc.addCourse1(list);
var c2 = mc.addCourse2(list);
System.out.print(c1 + " " + c2);
}
}
A:Javapython Javapython
B:JJavaJPython JavaPython
C:A RuntimeException is thrown
D:JJavaPython JavaPython
E:Compilation fails
ANSWER: Choice B
Topic:Using Object-Oriented Concepts in Java
QUESTION: 19
Which action enables the below code to compile?
class Course {
String name;
public static void getName() {
name = "Java";
System.out.println(name);
}
public void getName(String name) {
this.name += name;
System.out.println(name);
}
}
public class MyExamCloud {
public static void main(String[] args) {
var c = new Course();
c.getName("Python");
}
}
A:Replace String name; with final String name;
B:Replace String name; with static String name;
C:Replace public static void getName() with public void getName()
D:Replace public void getName(String name) with void getName(String name)
ANSWER: Choice B
Topic:Implement Localization
QUESTION: 20
Given:
app.properties file:
name = myexamcloud
app_en.properties file:
name = MyExamCloud(EN)
app_US.properties file:
name = MyExamCloud(US)
app_en_US.properties file:
name = MyExamCloud(EN US)
and the code fragment:
Locale.setDefault(Locale.US);
Locale currentLocale = new Locale.Builder().setLanguage("en").build();
ResourceBundle captions = ResourceBundle.getBundle("app.properties", currentLocale);
System.out.println(captions.getString("name"));
What is the result?
A:MyExamCloud(EN)
B:myexamcloud
C:MyExamCloud(US)
D:MyExamCloud(EN US)
E:Throws MissingResourceException
ANSWER: Choice B
Topic:Controlling Program Flow
QUESTION: 21
Code Given:
class MyExamCloud {
static void testName(Object o) {
switch (o) {
case null, String s -> System.out.println("Name: " + s.toString());
case
default -> System.out.println("Default");
}
}
public static void main(String as[]) {
testName(null);
}
}
What will happen when you compile and run the above code?
A:The code will not compile, we cannot have two combined cases (case null, String s)
B:The code will compile without any error and prints Name when run
C:The code will compile without any error and throws NullPointerException at runtime
D:The code will compile without any error and print Default.
ANSWER: Choice A
Topic:Handling Exceptions
QUESTION: 22
Given
1. class MyExamCloud {
2. public static void main(String args[]) {
3. try {
4. new MyExamCloud().method();
5. } catch(ArithmeticException e){
6. System.out.print("Arithmetic");
7. } finally {
8. System.out.print("final 1");
9. } finally {
10. System.out.print("final 2");
11. }
12. }
13.
14. public void method()throws ArithmeticException{
15. for(var x=0;x<5;x++){
16. var y = (int)5/x;
17. System.out.print(x);
18. }
19. }
20. }
What is the output?
A:Arithmetic
B:Arithmetic final 1
C:Arithmetic final 2
D:Arithmetic final 1 final 2
E:Compilation fails.
ANSWER: Choice E
Author | JEE Ganesh | |
Published | 7 months ago | |
Category: | Java Certification | |
HashTags | #Java #JavaCertification |