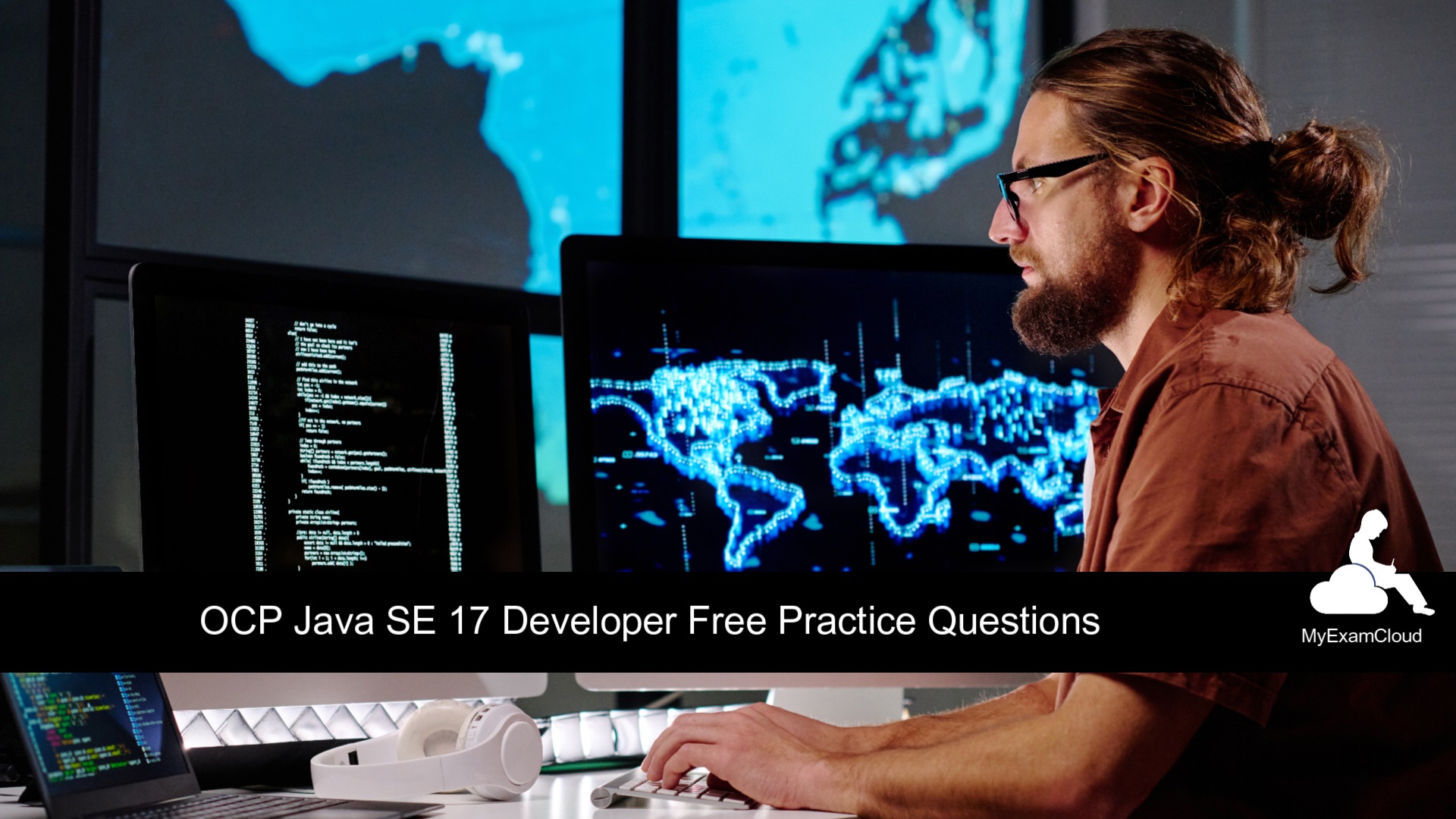
OCP Java 17 Developer 1Z0-829 Free Practice Questions
Prepare for the Oracle Certified Professional: Java SE 17 Developer certification exam with these practice questions. By earning this certification, you will demonstrate your proficiency in Java development and gain recognition from numerous industries. You will also showcase your extensive knowledge of the Java programming language, coding practices, and the latest features in Java SE 17.
These practice questions are based on the 1Z0-829 Practice Tests Study Plan by MyExamCloud, a comprehensive guide to assist you in your exam preparation. By completing these questions, you can become familiar with the exam format and content, and increase your chances of success.
To further enhance your understanding, the explanations provided in the 1Z0-829 Java SE 17 Developer Free Practice Mock Exam can serve as a valuable review of essential concepts for the exam.
Topic:Handing date, time, text, numeric and boolean values
QUESTION: 1
Code:
1. public class MyExamCloud{
2.
3. public static void main(String args[]){
4. int x = 10, y = 12;
5.
6. if( x++>11 & y-- > x){
7. System.out.println("passed");
8. } else{
9. System.out.println("failed");
10. }
11. }
12. }
Which of the following can be used to replace line 6?
A:if( Boolean.logicalAnd(x++>11 , y-- > x)){
B:if( Boolean.and(x++>11 , y-- > x)){
C:if( Boolean.or(x++>11 , y-- > x)){
D:if( Boolean.combine(x++>11 , y-- > x)){
ANSWER: Choice A
Topic:Handing date, time, text, numeric and boolean values
QUESTION: 2
Code:
1. class MyExamCloud {
2.
3. public static void main(String[] args){
4. var x = 1;
5. var y = 2;
6. System.out.print("" + x + y + " ");
7. System.out.print( x + y + " ");
8. System.out.print(x + " " + y);
9. }
10. }
Which is the output?
A:3 3 3
B:12 3 1 2
C:3 3 12
D:12 12 3
E:Compilation fails.
ANSWER: Choice B
Topic:Utilizing Java Object-Oriented Approach
QUESTION: 3
Code:
sealed interface MyInterface permits MyClass {}
non-seald MyClass implements MyInterfcace{}
Which of the following method can be inserted in to MyInterface?
A:void int getSpeed(){return 10;}
B:static int getSpeed() { return 10; }
C:default int getSpeed();
D:public default int getSpeed() { return 10; }
E:static abstract int getSpeed();
ANSWER: Choice B,Choice D
Topic:Utilizing Java Object-Oriented Approach
QUESTION: 4
Consider following three statements.
I. The variables declared inside a method are called as class variables.
II. The class variables are initialized to its default value.
III. The variable "s" in the declaration "short s = 10" should be an instance variable.
Which is/are true?
A:Only I.
B:Only II.
C:Only III.
D:Only I and II.
E:Only I and III.
ANSWER: Choice B
Topic:Utilizing Java Object-Oriented Approach
QUESTION: 5
Which of the following is a valid static method that can be used in an interface?
A:static double calc(int i, int j){ return i*j; }
B:protected static double calc(int i, int j){ return i*j; }
C:public abstract static double calc(int i, int j);
D:final static double calc(int i, int j){ return i*j; }
E:None of above.
ANSWER: Choice A
Topic:Handling Exceptions
QUESTION: 6
Given
1. class MyExamCloud{
2. public static void main(String args[]){
3. try{
4. new Program().method();
5. }catch(ArithmeticException e){
6. System.out.print("Arithmetic");
7. }finally{
8. System.out.print("final 1");
9. }finally{
10. System.out.print("final 2");
11. }
12. }
13.
14. public void method()throws ArithmeticException{
15. for(var x=0;x<5;x++){
16. var y = (int)5/x;
17. System.out.print(x);
18. }
19. }
20. }
What is the output?
A:Arithmetic
B:Arithmetic final 1
C:Arithmetic final 2
D:Arithmetic final 1 final 2
E:Compilation fails.
ANSWER: Choice E
Topic:Use Java I/O API
QUESTION: 7
Given
1. import java.io.IOException;
2. import java.nio.charset.Charset;
3. import java.nio.file.Files;
4. import java.nio.file.Paths;
5. import java.util.ArrayList;
6. import java.util.List;
7. import java.util.stream.Stream;
8.
9. public class MyExamCloud{
10. public static void main(String[] args) throws IOException{
11. var lines = new ArrayList<>();
12. var stream = Files.lines(Paths.get("MyTest.txt"), Charset.defaultCharset());
13. stream.map(String::trim).filter(s -> !s.isEmpty()).forEach(lines::add);
14. System.out.println(lines);
15. }
16. }
Content of the myTest.txt :
0 -> 1234
1 -> 1315
4 -> 1134
0 -> 4254
4 -> 9865
What is the output?
A:[0 -> 1234, 1 -> 1315, 4 -> 1134, , 0 -> 4254, 4 -> 9865]
B:[0 -> 1234, 1 -> 1315, 4 -> 1134]
C:[0 -> 1234, 1 -> 1315, 4 -> 1134, 0 -> 4254, 4 -> 9865]
D:Compilation fails due to error at line 12.
E:Compilation fails due to error at line 13.
ANSWER: Choice C
Topic:Access databases using JDBC
QUESTION: 8
Consider following steps
- Create a statement.
- Establishing a connection.
- Execute the query.
- Close the connection
- Process the ResultSet object.
What is the correct order of above steps to process any SQL statement with JDBC?
A:1,2,3,4,5
B:2,1,3,5,4
C:1,2,3,5,4
D:2,1,3,4,5
E:2,5,1,3,4
ANSWER: Choice B
Topic:Implement Localization
QUESTION: 9
Given
import java.util. * ;
public class MyExamCloud {
public static void main(String args[]) {
var ENUS = new Locale.Builder().setLanguage("en").setRegion("US").build();
System.out.print(ENUS.getDisplayCountry(new Locale("fr")));
}
}
What is the output?
A:Code will print the word "English" in English language (English).
B:Code will print the word "english" in French language (anglais).
C:Code will print the word "anglais" in English language (English).
D:Code will print the word "french" in French language (french).
E:Code will print the word "United States" in French language (Etats-Unis).
ANSWER: Choice E
Topic:Working with Streams and Lambda expressions
QUESTION: 10
Code:
1. import java.util.stream.DoubleStream;
2.
3. public class MyExamCloud {
4.
5. public static void main(String[] args){
6.
7. var doubles = DoubleStream.of(0.45,0.42,0.49);
8. System.out.print(doubles.filter(e -> e>0.45).count());
9. }
10. }
What is the output?
A:1
B:2
C:Compilation fails since we haven't imported predicate
D:Compilation fails due to error at line 7
E:Compilation fails due to error at line 8
ANSWER: Choice A
Topic:Working with Streams and Lambda expressions
QUESTION: 11
Consider:
IntStream stream = IntStream.of(2, 4, 6, 1, 34, 5, 9);
Code:
stream.average()
Which of the following above statement will return?
A:Double wrapper
B:double
C:int
D:OptionalDouble
E:None of above
ANSWER: Choice D
Topic:Working with Streams and Lambda expressions
QUESTION: 12
Code:
1. import java.util.stream.IntStream;
2.
3. public class MyExamCloud{
4. public static void main(String[] args){
5. var in = IntStream.range(3, 8);
6. var ins = in.peek(System.out::print).map(x -> x*2);
7. System.out.println(ins.noneMatch(x -> x%2 !=0));
8. }
9. }
What is the output?
A:34567false
B:68101214true
C:34567true
D:3false
E:Compilation fails.
ANSWER: Choice C
Topic:Package and deploy Java code and use the Java Platform Module System
QUESTION: 13
Given that a class named com.myexamcloud.exam.Exam is part of a module named com.myexamcloud.exam packaged in myexam.jar, which of the following commands can be used to execute this class?
(Assume that the jar file is located in the current directory.)
A:java --module-path myexam.jar --module com.myexamcloud.exam.Exam
B:java --module-path myexam.jar --module com.myexamcloud.exam com.myexamcloud.exam.Exam
C:java --module-path myexam.jar --module com.myexamcloud.exam --main-class com.myexamcloud.exam.Exam
D:java --module-path myexam.jar --module com.myexamcloud.exam/com.myexamcloud.exam.Exam
E:java --module-path . --module com.myexamcloud.exam/com.myexamcloud.exam.Exam
ANSWER: Choice D,Choice E
Topic:Manage concurrent code execution
QUESTION: 14
Consider the following code:
record Account(String accNumber, double balance) {
static java.util.concurrent.locks.ReentrantLock lock = new java.util.concurrent.locks.ReentrantLock();
public double withdraw(double amt) {
double latestBalance = 0;
try {
lock.lock();
if (balance > amt) latestBalance = balance - amt;
} finally {
lock.unlock();
}
return latestBalance;
}
}
What can be done to make the above code thread safe?
A:Change new ReentrantLock() to new ReentrantLock(true).
B:Make the lock variable as protected.
C:Make the lock variable protected, final, and static.
D:Move the call to lock.lock(); to before the try block.
E:Make the lock variable final.
F:No change is required.
G:Declare lock variable as private and final.
ANSWER: Choice G
Topic:Manage concurrent code execution
QUESTION: 15
Which of the following code fragments will you use to create an ExecutorService?
A:.getSingleThreadExecutor();
B:.createSingleThreadExecutor();
C:Executors
D:.newSingleThreadExecutor();
E:.newThreadExecutor();
F:Executor
G:ExecutorService
ANSWER: Choice C,Choice D
Topic:Use Java I/O API
QUESTION: 16
Given:
import java.io.*;
class MyExamCloud {
public static void main(String[] args) throws Exception {
try (var bfr = new BufferedReader(new InputStreamReader(System.in))) {
System.out.println("Enter Your Name:");
var s = bfr.readLine();
System.out.println("Your Name is : " + s);
} catch (Exception e) {
e.printStackTrace();
}
}
}
What will be the output if the above code is executed using the following command:
java MyExamCloud Joe
A:Enter Your Name:
Your Name is: MyExamCloud Joe
B:An exception stack trace will be printed.
C:Enter Your Name:
Your Name is : null
D:Enter Your Name:
E:Enter Your Name:
Your Name is : Joe
ANSWER: Choice D
Topic:Access databases using JDBC
QUESTION: 17
Given:
Connection con = DriverManager.getConnection(dbURL);
con.setAutoCommit(false);
String updateSQL =
"update ORDER " +
"set ORDER_AMOUNT = 99 where ORDER_EMAIL = 'pop@someemail.com'";
Statement stmt = con.createStatement();
stmt.executeUpdate(updateSQL);
//INSERT CODE HERE
What statement can be added to the above code so that the update is committed to the database?
A:stmt.commit();
B:stmt.commit(true);
C:con.commit(true);
D:con.setRollbackOnly(false);
E:con.setAutoCommit(true);
F:No code is necessary.
ANSWER: Choice E
Topic:Controlling Program Flow
QUESTION: 18
Code Given:
class MyExamCloud {
static void testName(Object o) {
switch (o) {
case null, String s -> System.out.println("Name: " + s.toString());
case
default -> System.out.println("Default");
}
}
public static void main(String as[]) {
testName(null);
}
}
What will happen when you compile and run the above code?
Assume that you have enabled the preview feature during compile time and runtime.
A:The code will not compile, we cannot have two combined cases (case null, String s)
B:The code will compile without any error and prints Name when run
C:The code will compile without any error and throws NullPointerException at runtime
D:The code will compile without any error and print Default.
ANSWER: Choice C
Topic:Controlling Program Flow
QUESTION: 19
Which of the following Java SE 17 code snippets compile without any error?
The variable obj is of type java.lang.Object.
Assume that you have enabled the preview feature during compile time and runtime.
A:
if (obj instanceof String name & name.length() > 10) {
var xPosition = 10;
var yPosition = 0;
}
B:
if (obj instanceof String name && name.length() > 10) {
var xPosition = 10;
var yPosition = 0;
}
C:
if (obj instanceof String name | name.length() > 10) {
var xPosition = 10;
var yPosition = 0;
}
D:
None of above
ANSWER: Choice B
Topic:Controlling Program Flow
QUESTION: 20
Code Given:
public boolean equals(Object o) {
String name = "Joe";
return (o instanceof String) &&
((String) o).equalsIgnoreCase(name);
}
Which of the following Java SE 17 code snippet replace the above?
Assume that you have enabled the preview feature during compile time and runtime.
A:public boolean equals(Object o) {
String name ="Joe";
return (o instanceof String is) ||
is.equalsIgnoreCase(name);
}
B:public boolean equals(Object o) {
String name ="Joe";
return (o instanceof String) &&
o.equalsIgnoreCase(name);
}
C:public boolean equals(Object o) {
String name ="Joe";
return (o instanceof Object) &&
((String)o).equalsIgnoreCase(name);
}
D:public boolean equals(Object o) {
String name ="Joe";
return (o instanceof String is) &&
is.equalsIgnoreCase(name);
}
ANSWER: Choice D
Topic:Controlling Program Flow
QUESTION: 21
Code Given:
//Saved as MyExamCloud.java
class Account {}
class StandardAccount extends Account {}
class PremiumAccount extends Account {
int monthsRemaining() {
return 10;
}
}
public class MyExamCloud {
record Point(int i, int j) {}
static void testType(Object o) {
switch (o) {
case null -> System.out.println("null");
case String s -> System.out.println("String");
case StandardAccount a -> System.out.println("Account");
case PremiumAccount p -> System.out.println("PremiumAccount");
case int[] ia -> System.out.println("Array");
default -> System.out.println("Something else");
}
}
public static void main(String as[]) {
testType(new PremiumAccount());
testType(new StandardAccount());
testType(new Account());
testType(null);
testType("");
testType(new int[] {
1, 2, 3, 4, 5
});
}
}
What is not true about MyExamCloud.java when you compile and run the above code?
Assume that you have enabled the preview feature during compile time and runtime.
A:The code may print both Account and PremiumAccount
B:The code will never print Something else
C:The code may print both Array and Something else
D:The code may print null and String
ANSWER: Choice B
Topic:Controlling Program Flow
QUESTION: 22
Code Given:
switch (o) {
case null:
default:
System.out.println("The rest (including null)");
}
What will happen when you compile and run the code by passing a null value?
Assume that you have enabled the preview feature during compile time and runtime.
A:The code will compile and print The rest (including null)
B:The code will not compile.
C:The code will compile and print nothing
D:NullPointerException will be thrown
ANSWER: Choice A
Topic:Controlling Program Flow
QUESTION: 23
Code:
class Exam {
public String toString() {
return "MyExamCloud Practice Exam";
}
}
class MyExamCloud {
public static void main(String as[]) {
Object o = new Exam();
switch (o) {
case null -> System.out.println("null");
case String s -> System.out.println("String");
case Exam e -> System.out.println(e.toString());
case int[] ia -> System.out.println("Array length" + ia.length);
default -> System.out.println("Something else");
}
}
}
What is the output?
Assume that you have enabled the preview feature during compile time and runtime.
A:@Exam
B:String
C:Array length 0
D:null
E:MyExamCloud Practice Exam
F:Something else
ANSWER: Choice E
Topic:Utilizing Java Object-Oriented Approach
QUESTION: 24
Code Given:
record Customer(Account account, int customerId) implements java.io.Serializable { // Line 1
public int customerId() {
return this.customerId + 1; // Line 3
}
public void someMethod() { // Line 4
System.out.println("Some method");
}
public static void test() { // Line 5
}
static {
System.out.println("A static intilizer record "); // Line 6
}
record InnerRecord() {} // Line 7
}
What is true about Customer record class?
A:Compiler error at // Line 5, we cannot have static methods
B:Compiler error at // Line 6, we cannot have static intilizer blocks
C:This type of record class should be considered bad style
D:Compiler error // Line 4, we cannot have additional instance methods
E:Compiler error at // Line 3, customerId is private final
F:Compiler error at // Line 7, we cannot have inner records
ANSWER: Choice C
Topic:Utilizing Java Object-Oriented Approach
QUESTION: 25
Code Given:
interface MyInterface {}
sealed class MyClass permits MyExamCloud {}
public final class MyExamCloud extends MyClass {
public static void main(String as[]) {
MyClass mc = new MyClass();
if (mc instanceof MyInterface)
System.out.println("MyInterface");
}
}
What will happen when you try to compile and run the above code?
A:Runtime exception will be thrown
B:Compile and print nothing
C:Compile and print nothing
D:Compile and print MyInterface
E:The code will not compile.
ANSWER: Choice E
Topic:Package and deploy Java code and use the Java Platform Module System
QUESTION: 26
Given
1. import java.time.MonthDay;
2. import java.time.Year;
3. import java.time.YearMonth;
4.
5. public class MyExamCloud{
6. public static void main(String[] args){
7. var y = Year.of(2015);
8. var ym = y.atMonthDay(MonthDay.of(2,2));
9. System.out.println(ym);
10. }
11. }
What is the output?
A:2015-02-02
B:2014-02-02
C:2014-01-01
D:An Exception is thrown.
E:Compilation fails.
ANSWER: Choice E
Topic:Controlling Program Flow
QUESTION: 27
Given the following options.
Which of the choices will fail to compile?
Assume that you have enabled the preview feature during compile time and runtime.
A:class Account {}
class StandardAccount extends Account {}
class PremiumAccount extends Account {
int monthsRemaining() {
return 10;
}
}
switch (a) {
case null:
break;
case PremiumAccount p:
if (p.monthsRemaining() >= 1) {
System.out.println("Subscription valid");
break;
}
}
B:static void test(Object o) {
switch (o) {
case String s -> System.out.println("String: " + s);
case int[] i -> System.out.println("Integer");
default -> System.out.println("default");
}
}
C: static int test(Object a) {
return switch (a) {
case int i -> 1;
case double d -> 3;
};
}
D:sealed class Account {}
final class StandardAccount extends Account {}
final class PremiumAccount extends Account {
int monthsRemaining() {
return 10;
}
}
Account a = new StandardAccount();
switch (a) {
case PremiumAccount p && (p.monthsRemaining() >= 1) ->
System.out.println("Subscription valid");
default ->
System.out.println("An Account, possibly a StandardAccount");
}
E: Object o = ...
switch (o) {
case null -> System.out.println("null");
case String s -> System.out.println("String");
case Exam e -> System.out.println(e.toString());
case char c -> System.out.println("Char" + c);
default -> System.out.println("Something else");
}
F:static int test(Object a) { return switch (a) { case Integer i -> 1; case Double d -> 3; default -> -1; }; }
and call to this method
int ret = test(123);
G:switch (o) {
case null:
default:
System.out.println("The rest (including null)");
}
ANSWER: Choice A,Choice C,Choice E
Topic:Handing date, time, text, numeric and boolean values
QUESTION: 28
Given the following options.
Which of the choices will fail to compile?
A:String myArticle =
"""
Java SE 17 is Good.
MyExamCloud Tests are realy useful
for latest Java Certifications. " """;
B:String type = ...
String code = String.format("""
public void print(%s o) {
System.out.println(Objects.toString(o));
}
""", type);
C:String code =
"""
String text = \"""
A text block inside a text block
\""";
""";
D:String myQuote =""" Pain is the healing process.
Failue is the success process.""";
ANSWER: Choice D
Topic:Utilizing Java Object-Oriented Approach
QUESTION: 29
Given the following options.
Which of the choices will fail to compile?
A:record MyRecord() extends MyClass {}
B:record MyRecord(String name, String address) {
static String welcome;
}
C:record Customer(int id, String email) {
Customer {
if (id<0)
throw new IllegalArgumentException(String.format("(%d,%s)", acN, email));
}
D:class MyClass {
class Inner {
static int n = 0; // Line 3
record Test() {} // Line 4
record Record(String n) {} // Line 5
}
}
E:record MyRecord(String name, String address) {
MyRecord record;
}
ANSWER: Choice A,Choice E
Topic:Utilizing Java Object-Oriented Approach
QUESTION: 30
Given the following options.
Which of the choices compile without any error?
A:record MyRecord() extends java.lang.Record {}
B:record MyRecord() extends MyClass {}
C:record MyRecord() implements MyInterface {}
D:class MyClass {
class Inner {
static int n = 0; // Line 3
record Test() {} // Line 4
record Record(String n) {} // Line 5
}
}
ANSWER: Choice C,Choice D
Topic:Working with Streams and Lambda expressions
QUESTION: 31
Given
1. //Assume all necessary importing have done
2.
3. public class MyExamCloud {
4. public static void main(String[] args){
5.
6. ArrayList<Double> list = new ArrayList<>();
7. list.add(21.6);
8. list.add(21.39);
9. list.add(21.5d);
10. list.add(21.41);
11.
12. //insert here
13.
14. for(Double d : list){
15. if(ip.test(d)){
16. System.out.println(d);
17. }
18. }
19. }
20. }
Which insert at line 12, will provide following output?
A:IntPredicate ip = i -> i > 21.4;
B:UnaryOperator<Double> ip = (i) ->{return i > 21.4;};
C:Consumer<Double> ip = (i) ->{return i > 21.4;};
D:Predicate ip = (i) -> {return i > 21.4;};
E:DoublePredicate ip = i -> i > 21.4;
ANSWER: Choice E
Topic:Working with Streams and Lambda expressions
QUESTION: 32
Given
1. import java.util.stream.Stream;
2.
3. public class MyExamCloud{
4. public static void main(String[] args){
5. Stream<String> stream = Stream.of("java","OCAJP");
6. System.out.println(stream.min((s1,s2) -> Integer.compare(s2.length(), s1.length())).get());
7. }
8. }
What is the output?
A:4
B:Java
C:OCAJP
D:An exception is thrown
E:Compilation fails
ANSWER: Choice C
Author | JEE Ganesh | |
Published | 7 months ago | |
Category: | Java Certification | |
HashTags | #Java #JavaCertification |