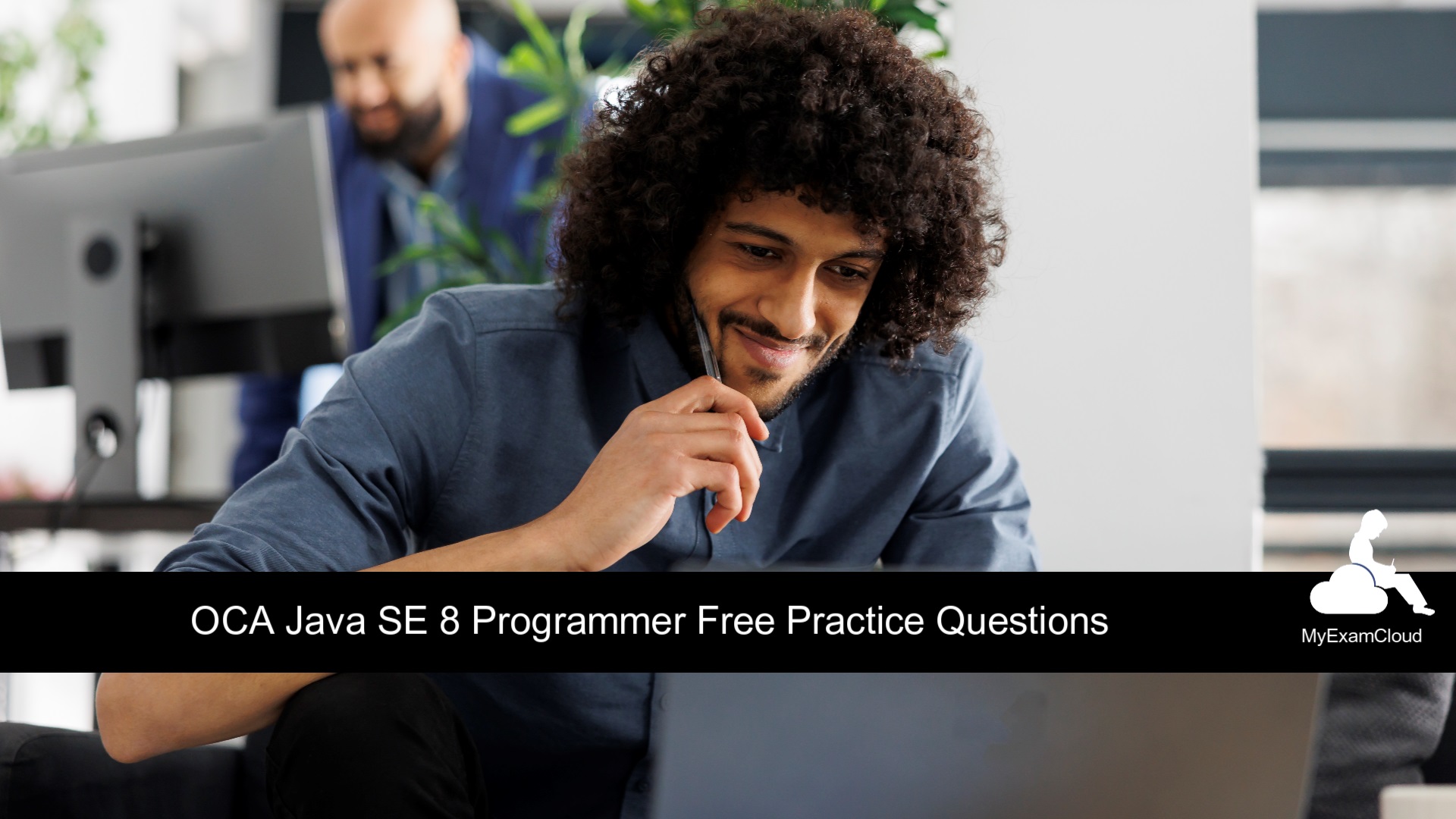
OCAJP 8 Free Practice Questions
These questions are based off of the OCAJP 8 Practice Tests Study Plan provided by MyExamCloud. The purpose of this practice plan is to help individuals prepare for the Oracle Certified Associate Java Programmer 8 certification exam. The exam tests the individual's knowledge and skills in programming concepts and the Java language. The following practice questions are selected from the study plan to help individuals practice and become familiar with the format and content of the actual exam.
Read brief explanations for the following questions from MyExamCloud OCAJP 8 Practice Tests Study Plan by taking Free 1Z0-808 Java SE 8 Programmer I Practice Mock Exam.
Topic:Java Basics
QUESTION:1
Code:
1. import java.util.Random;
2. import java.lang.System;
3. import java.util.*;
4. import java.lang.*;
5.
6. public class MyExamCloudOCAJP8 {
7.
8. public static void main(String[] args) {
9. Random r = new Random();
10. System.out.println(r.nextInt(10));
11.
12. }
13.}
Which lines contains redundant imports that not necessary for this code to compile?
A:Online lines 1 and 2
B:Online lines 1,2 and 3
C:Online lines 2,3 and 4
D:Online lines 1,3 and 4
E:All
ANSWER: Choice C
Topic:Java Basics
QUESTION:2
Which of the following can be use to run java code after compiling source code with file name, "MyFirst.java"?
A:javac MyFirst.class
B:java MyFirst.class
C:java MyFirst.java
D:javac MyFirst
E:java MyFirst
ANSWER: Choice E
Topic:Java Basics
QUESTION: 3
Which of the followings can be considered as enhancements in java 8?
I. Support for the Lambda Expression.
II. Multiple inheritances.
III. Default methods in abstract classes.
IV. Date and Time API
A:Only I.
B:Only III.
C:Only I and II.
D:Only I and IV.
E:Only I, III and IV.
ANSWER: Choice D
Topic:Java Basics
QUESTION:4
Code:
1. public class MyExamCloudOCAJP8 {
2.
3. public static void main(String[] a) {
4.
5. Integer arr[] = {1,2,3,4};
6. arr[1] = null;
7. for(Integer a : arr){
8. System.out.print(a);
9. }
10. }
11.}
What is the output?
A:1234
B:1024
C:1Null234
D:1 followed by a NullPointerException
E:Compilation fails.
ANSWER: Choice E
Topic:Java Basics
QUESTION:5
Which of the following is true about java source code files?
A:Each source file can contain only one class.
B:The file name must match the class name including case, and have a .java extension.
C:Each source file can contain only one public class.
D:All of above.
E:None of above.
ANSWER: Choice C
Topic:Working With Java Data Types
QUESTION:6
Which of the following is valid identifier?
A:2DPoint
B:$ocajp
C:new
D:java@elabs
E:$*coffee
ANSWER: Choice B
Topic:Creating and Using Arrays
QUESTION:7
Code:
1. public class MyExamCloudOCAJP8 {
2.
3. public static void main(String[] args){
4. Integer array[] = new Integer[0];
5. System.out.println(array[0]);
6. }
7. }
What is the output?
A:0
B:Null
C:NegativeArraySizeException
D:ArrayIndexOutOfBoundsException
E:Compilation fails.
ANSWER: Choice D
Topic:Working With Java Data Types
QUESTION:8
Code:
1. public class MyExamCloudOCAJP8 {
2.
3. static int y = 10;
4.
5. public static void main(String[] args){
6. int y;
7. System.out.println(y+MyExamCloudOCAJP8.y);
8. }
9. }
What is the output?
A:10
B:11
C:20
D:NullPointerException
E:Compilation error
ANSWER: Choice E
Topic:Working With Java Data Types
QUESTION:9
Code:
1. public class MyExamCloudOCAJP8 {
2. public static void main(String[] args) {
3. Integer one, two;
4. one = new Integer(1);
5. two = new Integer(2);
6. one = two;
7. one = null;
8. }
9. }
At line 8, which of the following/s eligible for garbage collection?
A:1 and 2
B:1 only
C:2 only
D:None of above
E:Compilation fails
ANSWER: Choice B
Topic:Working With Java Data Types
QUESTION:10
Code:
1. public class MyExamCloudOCAJP8{
2. public static void main(String[] args){
3. int x = 3;
4. int y = ++x * 4 / x-- + --x;
5. System.out.println("y + x is " + (y + x));
6. }
7. }
What is the output?
A:y + x is 7
B:y + x is 10
C:y + x is 9
D:y + x is 8
E:Compilation fails
ANSWER: Choice D
Topic:Using Loop Constructs
QUESTION:11
Code:
1. import java.util.ArrayList;
2. import java.util.List;
3.
4. public class MyExamCloudOCAJP8{
5.
6. public static void main(String[] args){
7. List<Integer> list = new ArrayList<Integer>();
8. list.add(1);
9. list.add(2);
10. list.add(3);
11. for(int x : list) {
12. System.out.print(x + " ");
13. break;
14. }
15. }
16.}
What is the output?
A:123
B:12
C:1
D:The code will not compile due to error at line 7
E:The code will not compile due to error at line 11
ANSWER: Choice C
Topic:Using Operators and Decision Constructs
QUESTION:12
Code:
1. public class MyExamCloudOCAJP8{
2. public static void main(String[] args){
3. String y = "Y";
4. final String n = "N";
5. String in = "y";
6.
7. switch(in.toUpperCase()){
8. case y : System.out.print("Yes");
9. case n : System.out.print("No");break;
10. default : System.out.print("Y/N");
11. }
12. }
13.}
What is the output?
A:Yes
B:No
C:YesNo
D:Y/N
E:The code will not compile due to error at line 8
ANSWER: Choice E
Topic:Working with Inheritance
QUESTION:13
Code:
public interface A{
void print();
}
Which of the following correctly override interface method A?
A:void print(){ System.out.println("A implemented"); }
B:public void print(){ System.out.println("A implemented"); }
C:String print(){ return "A implemented"; }
D:public String print(){ return "A implemented"; }
E:public abstract void print(){ System.out.println("A implemented"); }
ANSWER: Choice B
Topic:Working with Methods and Encapsulation
QUESTION:14
public void print(){
System.out.println("default");
}
Which of the following correctly overload above method?
A:private int print(int x){ return x; }
B:public String print(){ return "default"; }
C:public abstract void print(){ System.out.println("default"); }
D:void print(){ System.out.println("Non public"); }
E:None of above
ANSWER: Choice A
Topic:Working With Java Data Types
QUESTION: 15
Which of the following will convert following string to a primitive float?
String str = "1.2";
A:Float.parseFloat(str);
B:Float.valueOf(str);
C:Float.value(str);
D:Float.parse(str);
E:None of above
ANSWER: Choice A
Topic:Working with Methods and Encapsulation
QUESTION:16
Code:
1. package epractizelabs;
2.
3. public class A {
4. protected int y = 15;
5. }
6. package elab;
7.
8. import epractizelabs.A;
9.
10. public class B extends A{
11. int y = 10;
12. public void print(){
13. A a = new A();
14. System.out.println(a.y + y);
15. }
16. }
What is the output?
A:20
B:25
C:30
D:Compilation fails due to error at line 14
E:Compilation fails due to multiple errors
ANSWER: Choice D
Topic:Working with Methods and Encapsulation
QUESTION:17
Code:
1. public class A {
2.
3. int y;
4.
5. public A(int x){
6. y = x;
7. }
8.
9. public void print(){
10. System.out.println(y);
11. }
12. }
13.
14.
15. public class B extends A{
16. //constructor for this class
17. }
Which of the following can be used as the constructor for the class B?
A:public B(){ super(); }
B:public B(int x){ this(x); }
C:public B(int x){ System.out.println(x); super(x); }
D:public B(int x){ super(x); }
E:public A(){ super(); }
ANSWER: Choice D
Topic:Handling Exceptions
QUESTION:18
Code:
public static void main(String[] args){
Float number = Float.valueOf(args[0]);
}
Which of the following exceptions possible with above statement?
A:ParseException
B:ArrayIndexOutOfBoundsException
C:NumberFormatException
D:IOException
E:IllegalArgumentException
ANSWER: Choice B,Choice C
Topic:Working with Selected classes from the Java API
QUESTION:19
Which of the following will print current date and time?
A:System.out.print(new LocalTime().now());
B:System.out.print(new LocalTime());
C:System.out.println(Instant.now());
D:System.out.println(new Instant().now());
E:None of above.
ANSWER: Choice C
Topic:Working with Selected classes from the Java API
QUESTION:20
Code:
1. import java.time.LocalDate;
2. import java.time.Period;
3.
4. public class MyExamCloudOCAJP8 {
5. public static void main(String[] args) {
6. LocalDate date = LocalDate.of(2015, 3, 24);
7. Period p = Period.ofDays(2);
8. System.out.println(date.plus(p));
9. }
10.}
What is the output?
A:2015-04-26
B:2015-03-26
C:2015-02-27
D:Compilation fails due to error at line 6
E:Compilation fails due to error at line 8
ANSWER: Choice B
Topic:Working with Selected classes from the Java API
QUESTION:21
Code:
1. import java.time.LocalDate;
2.
3. public class MyExamCloudOCAJP8{
4. public static void main(String[] args) {
5. LocalDate date = LocalDate.parse("2014-12-30");
6. date = date.plusDays(2);
7. date.plusHours(12);
8. System.out.println(date.getYear() + " " + date.getMonth() + " " + date.getDayOfMonth());
9. }
10. }
What is the output?
A:2015 JANUARY 1
B:2015 JANUARY 2
C:2014 JANUARY 3
D:An exception is thrown
E:Compilation fails
ANSWER: Choice E
Topic:Working with Inheritance
QUESTION:22
Which of the following method can include in an interface?
A:static void print(){ System.out.println("interface"); }
B:static abstract void print();
C:default abstract void print();
D:default string toString(){ return "a"; }
E:None of above
ANSWER: Choice A
Topic:Working with Inheritance
QUESTION:23
Given
1. interface A{
2.
3. int groupID = 10;
4.
5. default boolean equals(Object obj){
6. return this.groupID == ((A)obj).groupID;
7. }
8.
9. static void print(){
10. System.out.println("A");
11. }
12.}
Which is true about above code?
A:Above code compiles fine
B:Code fails to compile since interface can't has any non abstract method
C:Compilation fails due to error at line 5
D:Compilation fails due to error at line 6
ANSWER: Choice C
Topic:Working with Inheritance
QUESTION:24
Given
1. public class MyExamCloudOCAJP8 {
2. public static void main(String[] args) {
3. Object numObj = new Integer(2);
4. String str = (String) numObj;
5. System.out.println(str);
6.
7. }
8. }
Which is true about above code?
A:2
B:Null
C:ClassCastException
D:IllegalArgumentException
E:NumberFormatException
ANSWER: Choice C
Topic:Working with Selected classes from the Java API
QUESTION:25
Consider following three statements
I. Function interface should contain only one method.
II. Function interface should contain only one method and it should be an abstract method.
III. Functional interface can have more than one method.
Which is/are true?
A:Only I
B:Only II
C:Only III
D:Only I and III
E:Only II and III
ANSWER: Choice C
Topic:Working with Selected classes from the Java API
QUESTION:26
Code:
1. import java.util.ArrayList;
2. import java.util.List;
3.
4. public class MyExamCloudOCAJP8{
5.
6. public static void main(String[] args){
7. List<Integer> list = new ArrayList<>();
8. list.add(1); list.add(11);
9. list.add(30); list.add(9);
10. list.removeIf(e -> e%2 != 0);
11. System.out.println(list);
12. }
13.}
Which is the output?
A:[1, 11, 9]
B:[30]
C:[]
D:Compilation fails due to error at line 7.
E:Compilation fails due to error at line 10.
ANSWER: Choice B
Topic:Working with Selected classes from the Java API
QUESTION:27
"You have a list orders of PurchaseOrder objects, each with a date, a Customer and a state. You want filter list in various ways"
Which of the following in built functional interface you are going to use for above?
A:UnaryOperator<T>
B:Consumer<T>
C:Supplier<T>
D:Predicate<T>
ANSWER: Choice D
Topic:Working with Selected classes from the Java API
QUESTION:28
Given
1. public interface A<R> extends B{
}
2. static void method(){
3. }
4. }
5.
6. public interface B<T> {
7. public void print(T t);
8.
9. static void print(){
10. }
11. }
12.
13. public interface C{
14. void methodC(String s);
15. }
16.
17. public interface D<T> extends A,B,C{
18. default void printer(T t){
19. }
20.
Which of the interfaces can be considered as functional interface/s?
A:Only interface A.
B:Only interface B.
C:Only interfaces A and B.
D:Only interfaces A, B and C.
E:All four interface are functional interface
ANSWER: Choice D
Topic:Working with Selected classes from the Java API
QUESTION:29
Consider following interface
interface Runnable{
public void run();
}
Which of the following will create instance of Runnable type?
A:Runnable run = () -> System.out.println("1Z0-808");
B:Runnable run = () -> { System.out.println("1Z0-808");}
C:Runnable run = new () -> System.out.println("1Z0-808");
D:Runnable run = > System.out.println("1Z0-808");
E:None of above
ANSWER: Choice A
Topic:Working with Selected classes from the Java API
QUESTION:30
Code:
1. public class MyExamCloudOCAJP8 {
2.
3. public static void main(String[] args){
4. String str = "1Z0";
5. StringBuilder sb = new StringBuilder("-");
6. sb.append("808");
7. str.concat(sb.toString());
8. System.out.println(str);
9. }
10.}
Which is the output?
A:1Z0
B:1Z0-808
C:An exception will be thrown
D:Compilation fails due to error at line 6
E:Compilation fails due to error at line 7
ANSWER: Choice A
Author | JEE Ganesh | |
Published | 7 months ago | |
Category: | Java Certification | |
HashTags | #Java #Programming |