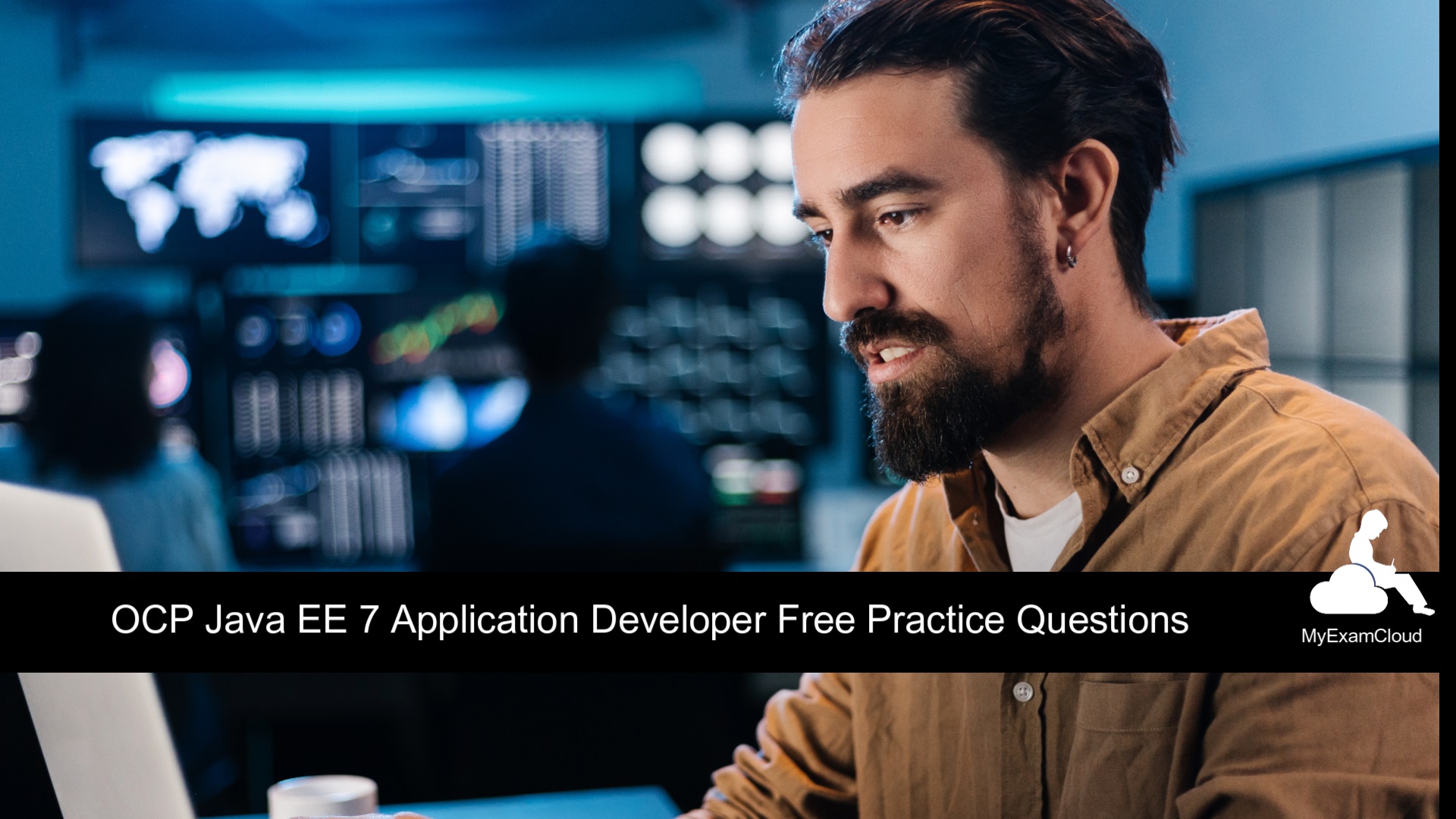
Java EE 7 Application Developer Free Practice Questions
The Oracle Certified Professional Java EE 7 Application Developer certification is for intermediate to advanced level application developers, architects, and software engineers who possess expertise in using Java EE 7 technology to create web-based user interfaces utilizing JavaScript, JSPs, JSFs, and servlets, as well as handling business logic. The certification exam, also known as 1Z0-900, aims to validate the candidate's programming skills in developing and deploying Java EE 7 applications.
These practice questions are designed to help individuals prepare for the certification exam by familiarizing themselves with the exam format and content. They are based on the 1Z0-900 Practice Tests Study Plan provided by MyExamCloud, which is a comprehensive guide for exam preparation. To get the most out of these practice questions, candidates are encouraged to take the 1Z0-900 Free Mock Exam available on MyExamCloud, which includes detailed explanations for each question. These explanations can help review key concepts and improve the chances of passing the certification exam.
Topic:Understand Java EE Architecture
QUESTION:1
Code:
@Stateless
@Local(CartSession.class)
public class PersistentCartSession implements CartSession {
@Override
public HashMap getCartItems(int userId) {
HashMap cartItems = new HasMap();
// logic to get cartItems
return cartItems;
}
}
@Stateless
@Local(CartSession.class)
public class TransientCartSession implements CartSession {
// code here
}
@WebServlet(name = "CartServlet", urlPatterns = { "/cart" })
public class CartServlet extends HttpServlet {
// code here
}
Which of the following code snippet inserted at // code here injects TransientCartSession in CartServlet?
A:
@EJB(beanName = "TransientCartSession")
CartSession session;
B:
@EJB
CartSession session;
C:
@EJB(type = "TransientCartSession")
CartSession session;
ANSWER: Choice A
Topic:Understand Java EE Architecture
QUESTION: 2
You are developing a Java EE application where most of the user interface page requires responsive HTML output. The page must populate navigation menu based on logged in role.
Which of the following Java EE web components can achieve this goal?
A:Java Servlet
B:JavaServer Faces
C:JavaServer Pages
D:CDI Beans
ANSWER: Choice A,Choice C
Topic:Manage Persistence using JPA Entities and BeanValidation
QUESTION: 3
Which of the following statements are not true about entities?
A:Entities support inheritance
B:Entities does not support polymorphic associations
C:Entities support polymorphic queries
D:Entities does not support polymorphic queries
ANSWER: Choice B,Choice D
Topic:Manage Persistence using JPA Entities and BeanValidation
QUESTION: 4
A developer working in EPractize Labs wants to fetch all customers whose order amount is greater than 500 USD. Assume that Customer entity is super class of PersonalCustomer and CorporateCustomer.
Which query can achieve this?
A:select c,p,d from Customer c, PersonalCustomer p, CorporateCustomer d where c.orderTotal > 500 AND p. orderTotal > 500 and d. orderTotal > 500
B:select c from Customer c where c.orderTotal > 500
C:select c,p,d from Customer c, PersonalCustomer p, CorporateCustomer d where c.orderTotal > 500
D:select c,p,d from Customer c, PersonalCustomer p, CorporateCustomer d where d.orderTotal > 500
ANSWER: Choice B
Topic:Implement Business Logic by Using EJBs
QUESTION: 5
Which statement is true about stateful session beans and stateless session beans?
A:Both stateful and stateless bean instances are required to survive container crashes.
B:Both stateful and stateless bean instances must be able to handle concurrent invocations from different threads.
C:A stateful bean with bean-managed transactions must commit or roll back any transaction before returning from a business method.
D:The container passivates and activates them using methods annotated with @PrePassivate and @PostActivate annotations for stateful session beans.
ANSWER: Choice D
Topic:Implement Business Logic by Using EJBs
QUESTION: 6
An EJB developer writes a CMT stateless session bean with one local business interface. All business methods are declared as REQUIRED. The bean has an injected field sessionCtx of the type SessionContext.
Which two operations are allowed in a business method of the bean?
A:sessionCtx.getEJBObject
B:sessionCtx.setRollbackOnly
C:sessionCtx.getMessageContext
D:sessionCtx.getBusinessObject
E:sessionCtx.getEJBLocalObject
ANSWER: Choice B,Choice D
Topic:Use Java Message Service API
QUESTION: 7
Which statement about message-driven beans is correct?
A:Each message-driven bean instance will be invoked only one thread at a time.
B:When dispatching messages to message bean instances the container must preserve the order in which messages arrive.
C:Each message-driven bean is associated with a JMS queue, each bean instance in the pool will receive each message sent to the queue.
D:If a message-driven bean is associated with a JMS durable subscription, each bean instance in the pool will receive each message sent to the durable subscription.
ANSWER: Choice A
Topic:Implement Business Logic by Using EJBs
QUESTION: 8
What is true about TimerService
interface?
A:The TimerService
interface is unavailable to stateless session beans.
B:The TimerService
interface is unavailable to stateful session beans.
C:The TimerService
interface is unavailable to singleton session beans.
ANSWER: Choice B
Topic:Use Java Message Service API
QUESTION: 9
Which of the following statements are true about Java EE 7 JMS message-driven bean with bean-managed transactions?
A:The message receipt is part of the user transaction.
B:Message acknowledgement is automatically handled by the container.
C:Messages are always processed in the order they were sent to the queue
D:Two messages read from the same queue may be processed at the same time within the same EJB container.
ANSWER: Choice B,Choice D
Topic:Implement SOAP Services by Using JAX-WS and JAXB APIs
QUESTION: 10
Which of the following session management mechanisms JAX-WS can support?
A:HTTP cookies
B:URL rewriting
C:SOAP based
D:Java Servlet HTTP Session
ANSWER: Choice A,Choice B,Choice C
Topic:Implement SOAP Services by Using JAX-WS and JAXB APIs
QUESTION: 11
Given in the below code snippet
@XmlType(propOrder = { "name", "address", "mobileNumber" })
//Code here
public class Customer {
@XmlElement(name = "Customer_Name", required = true)
public void setName(String name) {
this.name = name;
}
@XmlElement(name = "Customer_Address")
public void setAddress(String address) {
this.address = address;
}
@XmlAttribute(name = "mobileNumber", required = true)
public void setMobileNumber(String mobileNumber) {
this.mobileNumber = mobileNumber;
}
...
}
Which of the following JAXB marshal annotation convert Customer object as valid XML?
A:@XmlElement(name = "Customer")
B:@XmlRootElement(name = "Customer")
C:@XmlMainElement(name = "Customer")
ANSWER: Choice B
Topic:Create Java Web Applications using JSPs
QUESTION: 12
Which EL implicit object gives access to the parameters stored in ServletContext
object?
A:context
B:servletContext
C:applicationScope
ANSWER: Choice C
Topic:Create Java Web Applications using Servlets
QUESTION: 13
Code : A Servlet is mapped in the web.xml of the web application "order" as
<web-app>
...
<servlet-mapping>
<servlet-name>ManageProfile</servlet-name>
<url-pattern>ManageProfile</url-pattern>
</servlet-mapping>
...
<web-app>
Which of the following URLs would be served by this Servlet?
A:http://localhost/order/ManageProfile
B:http://localhost/order/manageprofile
C:http://localhost/order/manageProfile
D:None of the above
ANSWER: Choice D
Topic:Create Java Web Applications using JSPs
QUESTION:14
Given the JSP code:
<% request.setAttribute("orderNumber", "12345"); %>
and the Classic tag handler code:
8. public int doStartTag() throws JspException {
...
20. // some code here
21. }
Assume there are no other "orderNumber" attributes in the web application.
Which invocation on the pageContext object, inserted at line 9, assigns "12345" to the variable orderID?
A:String orderID = (String) pageContext.getAttribute("orderNumber");
B:String orderID = (String) pageContext.getRequestScope("orderNumber");
C:String orderID = (String) pageContext.getContextScope("orderNumber");
D:String orderID = (String) pageContext.getRequest().getAttribute("orderNumber");
ANSWER: Choice D
Topic:Create Java Web Applications using JSPs
QUESTION: 15
Which of the following syntax is used to embed a Java scriptlet in a JSP page?
A:<%@ ... %>
B:<% ... %>
C:<%= %>
D:<%! ... %>
ANSWER: Choice B
Topic:Implement REST Services using JAX-RS API
QUESTION: 16
Which of the following EJB's can use JAX-RS @Path annotation?
A:Stateless session bean
B:Stateful session bean
C:Singleton session bean
D:Message-driven Bean
ANSWER: Choice A,Choice C
Topic:Implement REST Services using JAX-RS API
QUESTION: 17
Given:
@Path("/customer/{id}")
public class Customer {
public Customer(@PathParam("id") String id) {
...
}
}
@Path("{lastname}")
public final class CustomerDetails {
...
}
A developer wants to convert this resource class scope as application.
Which CDI managed bean code can achieve this?
A:
@ApplicationScoped
@Path("/customer/{id}")
public class Customer {
public Customer(@PathParam("id") String id) {
...
}
}
@ApplicationScoped
@Path("{lastname}")
public final class CustomerDetails {
...
}
--
B
:@ApplicationScoped
@Path("/customer/{id}")
public class Customer {
public Customer(@PathParam("id") String id) {
...
}
}
@Path("{lastname}")
public final class CustomerDetails {
...
}
--
C:
@Path("/customer/{id}")
@ApplicationScoped
public class Customer {
public Customer() {
...
}
@Inject
public Customer(@PathParam("id") String id) {
...
}
}
@Path("{lastname}")
@ApplicationScoped
public class CustomerDetails {
...
}
ANSWER: Choice C
Topic:Create Java Applications using WebSockets
QUESTION: 18
A developer want to create a server endpoint to wait for text messages. Which of the following code can achieve this goal?
A:
public class MyWebSocket extends Endpoint {
@Override
public void onOpen(Session session, EndpointConfig ec) {
final RemoteEndpoint.Basic remote = session.getBasicRemote();
session.addMessageHandler(String.class, new MessageHandler.Whole < String > () {
public void onMessage(String text) {
try {
remote.sendText("Your message (" + text + ") has been recieved. Thanks !");
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
});
}
}
B:
@ServerEndpoint("/welcome")
public class MyWebSocket {
@OnMessage
public String handleMessage(String message) {
return "Your message (" + text + ") has been recieved. Thanks !";
}
}
C:
Both A and B
ANSWER: Choice C
Topic:Develop Web Applications using JSFs
QUESTION:19
Given code :
//index.xhtml
<h:commandLink action="examBoatSearch">
<h:outputText value="Proceed to ExamBoat Search Page"/>
</h:commandLink>
Which navigation rule correctly defines examBoatSearch?
Assume that ExamBoat search page is search.jsp.
A:
<navigation-rule>
<from-view-id>/index.xhtml</from-view-id>
<navigation-case>
<from-action>examBoatSearch</from-action>
<to-view-id>/search.jsp</to-view-id>
</navigation-case>
</navigation-rule>
B:
<navigation-rule>
<from-view-id>/index.xhtml</from-view-id>
<navigation-case>
<from-outcome>examBoatSearch</from-outcome>
<to-view-id>/search.jsp</to-view-id>
</navigation-case>
</navigation-rule>
C:
<navigation-rule>
<from-view-id>/index.xhtml</from-view-id>
<navigation-case>
<from-action>#{ManagedBean.actionMethod}</from-action>
<to-view-id>/search.jsp</to-view-id>
</navigation-case>
</navigation-rule>
D:None of the above, Both from-action and from-outcome are required.
ANSWER: Choice B
Topic:Create Java Applications using WebSockets
QUESTION: 20
@ServerEndpoint("/welcome")
public class MyWebSocket {
// Code here
public void handleNewConnection() {
System.out.print(“New connection recieved”);
}
@OnMessage
public String handleMessage(String message) {
return "Your message (" + text + ") has been recieved. Thanks !";
}
}
Which annotation inserted at // Code here will enabled handleNewConnection method must be called when there is a new connection?
A:@OnOpen
B:@OnClose
C:@OnNewConnection
D:@Init
ANSWER: Choice A
Topic:Develop Web Applications using JSFs
QUESTION: 21
Which JavaServer Faces life cycle will be started when a link or a button component is clicked?
A:Update Model Values Phase
B:Apply Request Values Phase
C:Invoke Application Phase
D:Restore View Phase
E:Render Response Phase
F:Process Validations Phase
ANSWER: Choice D
Topic:Secure Java EE 7 Applications
QUESTION: 22
Which of the following code defines login-config for Form-based authentication?
A:<login-config>
<auth-method>FORM</auth-method>
<realm-name>file</realm-name>
<form-login-config>
<form-login-page>/logon.jsp</form-login-page>
<form-error-page>/logonError.jsp</form-error-page>
</form-login-config>
</login-config>
B:<login-config>
<auth-method>FORM</auth-method>
<realm-name>file</realm-name>
<form-config>
<login-page>/logon.jsp</form-login-page>
<error-page>/logonError.jsp</form-error-page>
</form-config>
</login-config>
C:<login-config>
<auth-method>FORM</auth-method>
<realm>file</realm>
<form-login-config>
<form-login-page>/logon.jsp</form-login-page>
<form-error-page>/logonError.jsp</form-error-page>
</form-login-config>
</login-config>
D:<login-config>
<authentication-method>FORM</authentication-method>
<realm-name>file</realm-name>
<form-login-config>
<form-login-page>/logon.jsp</form-login-page>
<form-error-page>/logonError.jsp</form-error-page>
</form-login-config>
</login-config>
ANSWER: Choice A
Topic:Use CDI Beans
QUESTION: 23
Given the below CDI beans
// Customer bean
@Default
public class Customer {
...
}
// Product bean
public class Product {
...
}
How many qualifiers are defined Customer and product beans?
A:Customer bean has one qualifier and Product bean has none
B:Both Customer and Product bean has only one qualifier
C:Both Customer and Product bean has two qualifiers
D:Both Customer and Product bean has three qualifiers
ANSWER: Choice C
Topic:Use Concurrency API in Java EE 7 Applications
QUESTION: 24
public class ReportTask implements Runnable {
public int final NEW_REPORT = 1;
public int final OLD_REPORT = 2;
int reportType = NEW_REPORT;
public ReportTask() {}
public ReportTask(int reportType) {
this.reportType = reportType;
}
public void run() {
// Logic here
}
}
public class ReportServlet extends HttpServlet {
@Resource(name = "concurrent/MyAppTasksExecutor")
ManagedExecutorService mes;
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//Code here
}
}
Which of the following inserted at //code here correctly submit ReportTask to MyAppTasksExecutor?
A:ReportTask reportTask = new ReportTask();
reportTask.start();
B:ReportTask reportTask = new ReportTask();
Future reportFuture = mes.run(reportTask);
C:ReportTask reportTask = new ReportTask();
Future reportFuture = mes.submit(reportTask);
D:ReportTask reportTask = new ReportTask();
Future reportFuture = mes.join(reportTask);
ANSWER: Choice C
Topic:Use Batch API in Java EE 7 Applications
QUESTION: 25
The PayrollJob batch job involves reading input data for payroll processing from a XL sheet. Each line in the file contains an employee ID and the base salary (per month) for one employee. The batch job then calculates the tax to be withheld, the bonus, and the net salary. The job finally needs to write out the processed payroll records into a database table.
@Named
public class SimpleItemReader extends AbstractItemReader {
@Inject
private JobContext jobContext;
...
}
public class SimpleItemProcessor implements ItemProcessor {
@Inject
private JobContext jobContext;
public Object processItem(Object obj) throws Exception {
....
}
}
@Named
public class SimpleItemWriter extends AbstractItemWriter {
@PersistenceContext
EntityManager em;
public void writeItems(List list) throws Exception {
for (Object obj: list) {
System.out.println("PayrollRecord: " + obj);
em.persist(obj);
}
}
....
}
Which JSL correctly defines chunk-style step for these batch jobs?
A:<job id="PayrollJob" xmlns=http://xmlns.jcp.org/xml/ns/javaee version="1.0">
<step id="process">
<chunk item-count="2">
<reader ref="simpleItemReader/>
<processor ref="simpleItemProcessor/>
<writer ref="simpleItemWriter/>
</chunk>
</step>
</job>
B:<job id="PayrollJob" xmlns=http://xmlns.jcp.org/xml/ns/javaee version="1.0">
<step id="process">
<chunk item-count="2">
<reader class="SimpleItemReader/>
<processor class="SimpleItemProcessor/>
<writer class="SimpleItemWriter/>
</chunk>
</step>
</job>
C:<job id="PayrollJob" xmlns=http://xmlns.jcp.org/xml/ns/javaee version="1.0">
<step id="process">
<chunk item-count="2">
<reader name="simpleItemReader/>
<processor name="simpleItemProcessor/>
<writer name="simpleItemWriter/>
</chunk>
</step>
</job>
ANSWER: Choice A
Topic:Create Java Web Applications using JSPs
QUESTION: 26
Which of the following is the parent element of <error-page> element?
A:error-code
B:servlet
C:servlet-mapping
D:web-app
E:exception-type
ANSWER: Choice D
Topic:Implement SOAP Services by Using JAX-WS and JAXB APIs
QUESTION: 27
Assume that you have defined Customer class with valid JAXB marshal annotations.
Customer customer = new Customer();
customer.setName("Joe Pop");
customer.setAddress(“919 Smart Street Phase I, Gandhi Nagar, Chennai”);
customer.setMobileNumber(“9000000”);
Which of the following code initiate JAXB marshaller?
A:JAXBContext jaxbContext = JAXBContext.newInstance(Customer.class);
Marshaller jaxbMarshaller = jaxbContext.initMarshaller();
B:JAXBContext jaxbContext = JAXBContext.newInstance(Customer.class);
Marshaller jaxbMarshaller = jaxbContext.createMarshaller();
C:JAXBContext jaxbContext = JAXBContext.createCustomer.class);
Marshaller jaxbMarshaller = jaxbContext.createMarshaller();
ANSWER: Choice B
Topic:Create Java Web Applications using Servlets
QUESTION: 28
You are assigned to define servlet mappings in web.xml for the following classes.
com.epractizelabs.web.OrderServlet
com.epractizelabs.web.LicenseServlet
Which of the following web.xml fragments are correct?
A:<servlet>
<servlet-class>com.epractizelabs.web.OrderServlet</servlet-class>
<servlet-name>order</servlet-name>
</servlet>
<servlet>
<servlet-class>com.epractizelabs.web.LicenseServlet</servlet-class>
<servlet-name>license</servlet-name>
</servlet>
B:<servlet>
<servlet-name>order</servlet-name>
<servlet-class>com.epractizelabs.web.OrderServlet</servlet-class>
</servlet>
<servlet>
<servlet-name>license</servlet-name>
<servlet-class>com.epractizelabs.web.LicenseServlet</servlet-class>
</servlet>
C:<servlet>
<servlet-name>licenseServlet</servlet-name>
<servlet-class>com.epractizelabs.web.LicenseServlet.class</servlet-class>
</servlet>
<servlet>
<servlet-name>orderServlet</servlet-name>
<servlet-class>com.epractizelabs.web.OrderServlet.class</servlet-class>
</servlet>
D:<servlet>
<name>licenseServlet</name>
<class>com.epractizelabs.web.LicenseServlet<class>
</servlet>
<servlet>
<name>orderServlet</name>
<class>com.epractizelabs.web.OrderServlet<class>
</servlet>
ANSWER: Choice B
Author | JEE Ganesh | |
Published | 7 months ago | |
Category: | Java Certification | |
HashTags | #Java #JavaCertification |