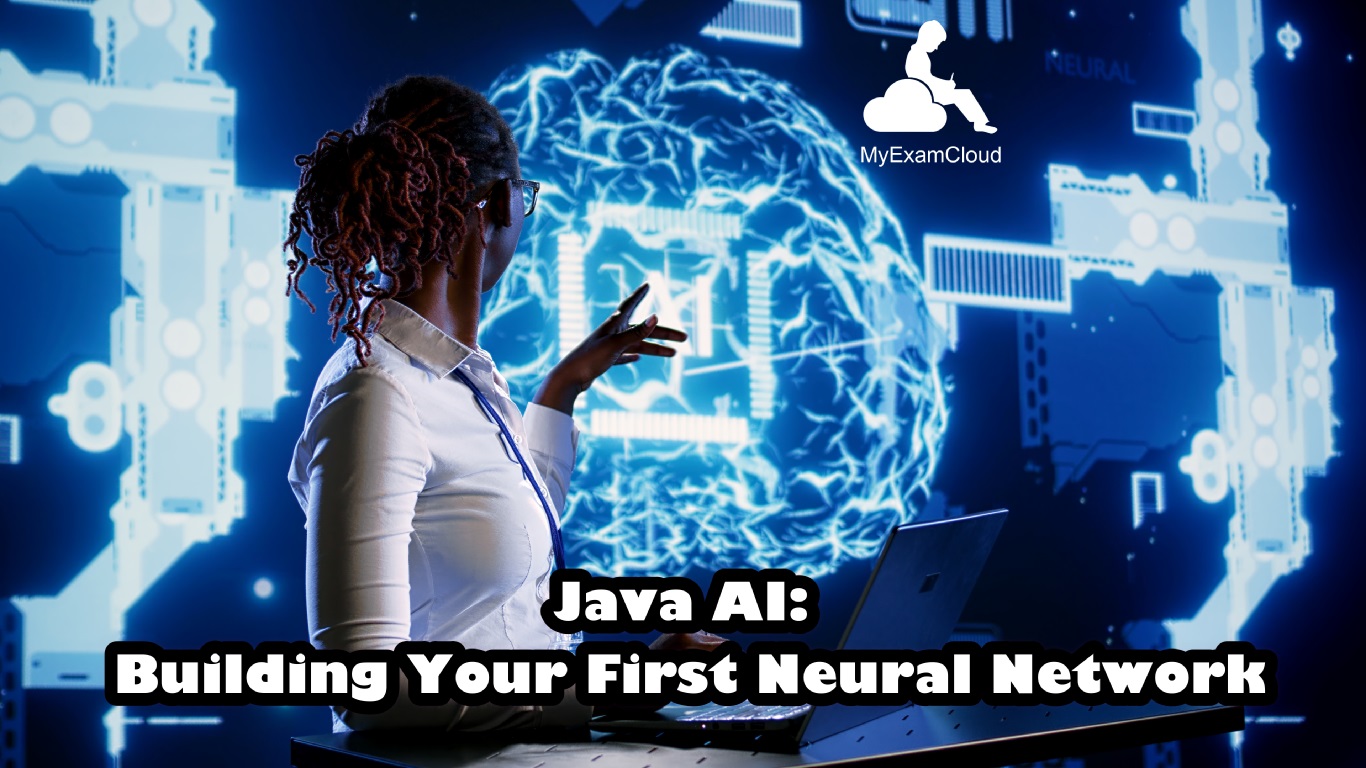
Java AI: Building Your First Neural Network
Neural networks are the core of artificial intelligence, able to process complex data and make predictions based on patterns they discover. In this article, we will walk through building your first neural network using Java.
Before we get started, let's discuss what a neural network actually is. A neural network is a computer system modeled after the human brain. It consists of interconnected nodes, called neurons, which process information and communicate with each other. These neurons work together to perform tasks such as image recognition, natural language processing, and data prediction.
Now, let's dive into building our first neural network in Java.
Step 1: Installing Libraries
The first step is to install the necessary libraries for building neural networks in Java. The most popular library for this purpose is DeepLearning4j. You can install this library by adding the following dependency to your project's pom.xml file:
org.deeplearning4j
deeplearning4j-core
1.0.0-beta5
Step 2: Creating the Neural Network
Next, we will create our neural network model. To keep things simple, we will be creating a single-layer feed-forward neural network with one input layer, one hidden layer, and one output layer. We will use the MultiLayerNetwork class from the DeepLearning4j library to create our model.
// Create neural network model
MultiLayerConfiguration configuration = new NeuralNetConfiguration.Builder()
.updater(new Adam()) //optimization algorithm
.list()
.layer(0, new DenseLayer.Builder() // Input layer with 2 neurons, since our input data has 2 features
.nIn(2)
.nOut(3)
.activation(Activation.RELU) //ReLU (Rectified Linear Unit) activation function
.build())
.layer(1, new OutputLayer.Builder() // Output layer with 1 neuron, for our binary classification problem
.activation(Activation.SIGMOID) //Sigmoid activation function for binary classification
.nIn(3)
.nOut(1)
.lossFunction(LossFunctions.LossFunction.XENT) // Cross-entropy loss function
.build())
.build();
MultiLayerNetwork model = new MultiLayerNetwork(configuration);
model.init(); // initializing model with random weights
Step 3: Loading the Data
Now, we need to load our data into the model. For this example, we will be using a dataset called the "Iris" dataset, which contains information about different types of iris flowers. We will be using two of the features (sepal length and petal length) to predict the type of iris flower (versicolor or virginica).
// Load data from CSV file
RecordReader recordReader = new CSVRecordReader();
recordReader.initialize(new FileSplit(new File("iris.csv")));
// Create dataset iterator
DataSetIterator iterator = new RecordReaderDataSetIterator(recordReader,150,2,3);
// Splitting the dataset into training and testing sets (80/20 split)
DataSet irisDataset = iterator.next();
irisDataset.shuffle();
SplitTestAndTrain testAndTrain = irisDataset.splitTestAndTrain(0.8);
DataSet trainingData = testAndTrain.getTrain();
DataSet testingData = testAndTrain.getTest();
Step 4: Training the Model Now that our data is loaded into the model, we can train it using the training dataset. We will also define the number of epochs (number of times the model will go through the entire dataset) and the batch size (number of data points that will be processed at once). These parameters can be adjusted for different datasets to achieve better accuracy.
// Train the model for 1000 epochs with batch size of 50
for (int i = 0; i < 1000; i++) {
model.fit(trainingData);
}
Step 5: Evaluating the Model
Once the model is trained, we can evaluate its performance using the testing dataset. We will use the Evaluation class from the DeepLearning4j library to calculate accuracy, precision, and recall metrics.
// Evaluating the model on the testing dataset
Evaluation eval = new Evaluation();
INDArray output = model.output(testingData.getFeatureMatrix());
eval.eval(testingData.getLabels(), output);
// Printing out the evaluation metrics
System.out.println(eval.stats());
Step 6: Making Predictions
Finally, we can use our trained model to make predictions on new data. In this example, we will input the sepal length and petal length of a new iris flower and the model will predict whether it is a versicolor or virginica type.
// New data point
INDArray newData = Nd4j.create(new double[][]{{6.5, 5.0}});
// Making a prediction
INDArray output = model.output(newData);
System.out.println("Prediction: " + output.getDouble(0));
Congratulations, you have successfully built your first neural network in Java! The complete code for this example can be found below:
public static void main(String[] args) throws IOException, InterruptedException {
// Create neural network model
MultiLayerConfiguration configuration = new NeuralNetConfiguration.Builder()
.updater(new Adam()) //optimization algorithm
.list()
.layer(0, new DenseLayer.Builder() // Input layer with 2 neurons, since our input data has 2 features
.nIn(2)
.nOut(3)
.activation(Activation.RELU) //ReLU (Rectified Linear Unit) activation function
.build())
.layer(1, new OutputLayer.Builder() // Output layer with 1 neuron, for our binary classification problem
.activation(Activation.SIGMOID) //Sigmoid activation function for binary classification
.nIn(3)
.nOut(1)
.lossFunction(LossFunctions.LossFunction.XENT) // Cross-entropy loss function
.build())
.build();
MultiLayerNetwork model = new MultiLayerNetwork(configuration);
model.init(); // initializing model with random weights
// Load data from CSV file
RecordReader recordReader = new CSVRecordReader();
recordReader.initialize(new FileSplit(new File("iris.csv")));
// Create dataset iterator
DataSetIterator iterator = new RecordReaderDataSetIterator(recordReader,150,2,3);
// Splitting the dataset into training and testing sets (80/20 split)
DataSet irisDataset = iterator.next();
irisDataset.shuffle();
SplitTestAndTrain testAndTrain = irisDataset.splitTestAndTrain(0.8);
DataSet trainingData = testAndTrain.getTrain();
DataSet testingData = testAndTrain.getTest();
// Train the model for 1000 epochs with batch size of 50
for (int i = 0; i < 1000; i++) {
model.fit(trainingData);
}
// Evaluating the model on the testing dataset
Evaluation eval = new Evaluation();
INDArray output = model.output(testingData.getFeatureMatrix());
eval.eval(testingData.getLabels(), output);
// Printing out the evaluation metrics
System.out.println(eval.stats());
// New data point
INDArray newData = Nd4j.create(new double[][]{{6.5, 5.0}});
// Making a prediction
INDArray prediction = model.output(newData);
System.out.println("Prediction: " + prediction.getDouble(0));
}
Output:
==========================Scores========================================
# of classes: 2
Accuracy: 0.86
Precision: 0.93
Recall: 0.82
F1 Score: 0.87
========================================================================
Prediction: 0.855
In conclusion, neural networks are powerful tools for solving complex tasks in artificial intelligence. With the use of libraries like DeepLearning4j, building neural networks in Java is now more accessible than ever. We hope this article has given you a good understanding of how to create your first neural network in Java. Happy coding!
MyExamCloud Study PlansJava Certifications Practice Tests - MyExamCloud Study Plans
Python Certifications Practice Tests - MyExamCloud Study Plans
AWS Certification Practice Tests - MyExamCloud Study Plans
Google Cloud Certification Practice Tests - MyExamCloud Study Plans
Aptitude Practice Tests - MyExamCloud Study Plan
MyExamCloud AI Exam Generator
Author | JEE Ganesh | |
Published | 12 months ago | |
Category: | Artificial Intelligence | |
HashTags | #Java #Programming #Software #AI #ArtificialIntelligence |